Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse
mssql.getAzureadServicePrincipal
Explore with Pulumi AI
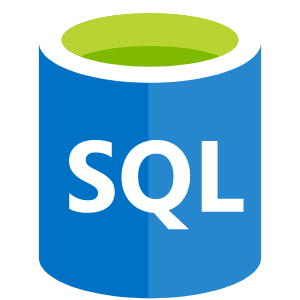
Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse
Obtains information about single Azure AD Service Principal database user.
Example Usage
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Mssql = Pulumi.Mssql;
return await Deployment.RunAsync(() =>
{
var exampleDatabase = Mssql.GetDatabase.Invoke(new()
{
Name = "example",
});
var exampleAzureadServicePrincipal = Mssql.GetAzureadServicePrincipal.Invoke(new()
{
Name = "example",
DatabaseId = exampleDatabase.Apply(getDatabaseResult => getDatabaseResult.Id),
});
return new Dictionary<string, object?>
{
["appClientId"] = exampleAzureadServicePrincipal.Apply(getAzureadServicePrincipalResult => getAzureadServicePrincipalResult.ClientId),
};
});
package main
import (
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
"github.com/pulumiverse/pulumi-mssql/sdk/go/mssql"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
exampleDatabase, err := mssql.LookupDatabase(ctx, &mssql.LookupDatabaseArgs{
Name: "example",
}, nil)
if err != nil {
return err
}
exampleAzureadServicePrincipal, err := mssql.LookupAzureadServicePrincipal(ctx, &mssql.LookupAzureadServicePrincipalArgs{
Name: pulumi.StringRef("example"),
DatabaseId: exampleDatabase.Id,
}, nil)
if err != nil {
return err
}
ctx.Export("appClientId", exampleAzureadServicePrincipal.ClientId)
return nil
})
}
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.mssql.MssqlFunctions;
import com.pulumi.mssql.inputs.GetDatabaseArgs;
import com.pulumi.mssql.inputs.GetAzureadServicePrincipalArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var exampleDatabase = MssqlFunctions.getDatabase(GetDatabaseArgs.builder()
.name("example")
.build());
final var exampleAzureadServicePrincipal = MssqlFunctions.getAzureadServicePrincipal(GetAzureadServicePrincipalArgs.builder()
.name("example")
.databaseId(exampleDatabase.applyValue(getDatabaseResult -> getDatabaseResult.id()))
.build());
ctx.export("appClientId", exampleAzureadServicePrincipal.applyValue(getAzureadServicePrincipalResult -> getAzureadServicePrincipalResult.clientId()));
}
}
import pulumi
import pulumi_mssql as mssql
example_database = mssql.get_database(name="example")
example_azuread_service_principal = mssql.get_azuread_service_principal(name="example",
database_id=example_database.id)
pulumi.export("appClientId", example_azuread_service_principal.client_id)
import * as pulumi from "@pulumi/pulumi";
import * as mssql from "@pulumi/mssql";
const exampleDatabase = mssql.getDatabase({
name: "example",
});
const exampleAzureadServicePrincipal = exampleDatabase.then(exampleDatabase => mssql.getAzureadServicePrincipal({
name: "example",
databaseId: exampleDatabase.id,
}));
export const appClientId = exampleAzureadServicePrincipal.then(exampleAzureadServicePrincipal => exampleAzureadServicePrincipal.clientId);
variables:
exampleDatabase:
fn::invoke:
Function: mssql:getDatabase
Arguments:
name: example
exampleAzureadServicePrincipal:
fn::invoke:
Function: mssql:getAzureadServicePrincipal
Arguments:
name: example
databaseId: ${exampleDatabase.id}
outputs:
appClientId: ${exampleAzureadServicePrincipal.clientId}
Using getAzureadServicePrincipal
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getAzureadServicePrincipal(args: GetAzureadServicePrincipalArgs, opts?: InvokeOptions): Promise<GetAzureadServicePrincipalResult>
function getAzureadServicePrincipalOutput(args: GetAzureadServicePrincipalOutputArgs, opts?: InvokeOptions): Output<GetAzureadServicePrincipalResult>
def get_azuread_service_principal(client_id: Optional[str] = None,
database_id: Optional[str] = None,
name: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetAzureadServicePrincipalResult
def get_azuread_service_principal_output(client_id: Optional[pulumi.Input[str]] = None,
database_id: Optional[pulumi.Input[str]] = None,
name: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetAzureadServicePrincipalResult]
func LookupAzureadServicePrincipal(ctx *Context, args *LookupAzureadServicePrincipalArgs, opts ...InvokeOption) (*LookupAzureadServicePrincipalResult, error)
func LookupAzureadServicePrincipalOutput(ctx *Context, args *LookupAzureadServicePrincipalOutputArgs, opts ...InvokeOption) LookupAzureadServicePrincipalResultOutput
> Note: This function is named LookupAzureadServicePrincipal
in the Go SDK.
public static class GetAzureadServicePrincipal
{
public static Task<GetAzureadServicePrincipalResult> InvokeAsync(GetAzureadServicePrincipalArgs args, InvokeOptions? opts = null)
public static Output<GetAzureadServicePrincipalResult> Invoke(GetAzureadServicePrincipalInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetAzureadServicePrincipalResult> getAzureadServicePrincipal(GetAzureadServicePrincipalArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: mssql:index/getAzureadServicePrincipal:getAzureadServicePrincipal
arguments:
# arguments dictionary
The following arguments are supported:
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Client
Id string - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- Name string
- User name. Cannot be longer than 128 chars.
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Client
Id string - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- Name string
- User name. Cannot be longer than 128 chars.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - client
Id String - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- name String
- User name. Cannot be longer than 128 chars.
- database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - client
Id string - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- name string
- User name. Cannot be longer than 128 chars.
- database_
id str - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - client_
id str - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- name str
- User name. Cannot be longer than 128 chars.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - client
Id String - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- name String
- User name. Cannot be longer than 128 chars.
getAzureadServicePrincipal Result
The following output properties are available:
- Client
Id string - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Id string
<database_id>/<user_id>
. User ID can be retrieved usingsys.database_principals
view.- Name string
- User name. Cannot be longer than 128 chars.
- Client
Id string - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Id string
<database_id>/<user_id>
. User ID can be retrieved usingsys.database_principals
view.- Name string
- User name. Cannot be longer than 128 chars.
- client
Id String - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id String
<database_id>/<user_id>
. User ID can be retrieved usingsys.database_principals
view.- name String
- User name. Cannot be longer than 128 chars.
- client
Id string - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id string
<database_id>/<user_id>
. User ID can be retrieved usingsys.database_principals
view.- name string
- User name. Cannot be longer than 128 chars.
- client_
id str - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- database_
id str - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id str
<database_id>/<user_id>
. User ID can be retrieved usingsys.database_principals
view.- name str
- User name. Cannot be longer than 128 chars.
- client
Id String - Azure AD client_id of the Service Principal. This can be either regular Service Principal or Managed Service Identity.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id String
<database_id>/<user_id>
. User ID can be retrieved usingsys.database_principals
view.- name String
- User name. Cannot be longer than 128 chars.
Package Details
- Repository
- mssql pulumiverse/pulumi-mssql
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
mssql
Terraform Provider.
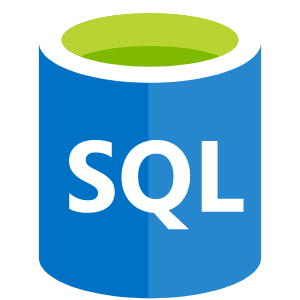
Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse