Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse
mssql.getSchema
Explore with Pulumi AI
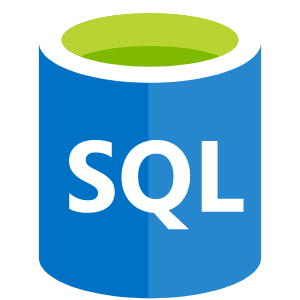
Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse
Retrieves information about DB schema.
Example Usage
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Mssql = Pulumi.Mssql;
return await Deployment.RunAsync(() =>
{
var example = Mssql.GetDatabase.Invoke(new()
{
Name = "example",
});
var byName = Mssql.GetSchema.Invoke(new()
{
DatabaseId = example.Apply(getDatabaseResult => getDatabaseResult.Id),
Name = "dbo",
});
});
package main
import (
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
"github.com/pulumiverse/pulumi-mssql/sdk/go/mssql"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
example, err := mssql.LookupDatabase(ctx, &mssql.LookupDatabaseArgs{
Name: "example",
}, nil)
if err != nil {
return err
}
_, err = mssql.LookupSchema(ctx, &mssql.LookupSchemaArgs{
DatabaseId: pulumi.StringRef(example.Id),
Name: pulumi.StringRef("dbo"),
}, nil)
if err != nil {
return err
}
return nil
})
}
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.mssql.MssqlFunctions;
import com.pulumi.mssql.inputs.GetDatabaseArgs;
import com.pulumi.mssql.inputs.GetSchemaArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var example = MssqlFunctions.getDatabase(GetDatabaseArgs.builder()
.name("example")
.build());
final var byName = MssqlFunctions.getSchema(GetSchemaArgs.builder()
.databaseId(example.applyValue(getDatabaseResult -> getDatabaseResult.id()))
.name("dbo")
.build());
}
}
import pulumi
import pulumi_mssql as mssql
example = mssql.get_database(name="example")
by_name = mssql.get_schema(database_id=example.id,
name="dbo")
import * as pulumi from "@pulumi/pulumi";
import * as mssql from "@pulumi/mssql";
const example = mssql.getDatabase({
name: "example",
});
const byName = example.then(example => mssql.getSchema({
databaseId: example.id,
name: "dbo",
}));
variables:
example:
fn::invoke:
Function: mssql:getDatabase
Arguments:
name: example
byName:
fn::invoke:
Function: mssql:getSchema
Arguments:
databaseId: ${example.id}
name: dbo
Using getSchema
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getSchema(args: GetSchemaArgs, opts?: InvokeOptions): Promise<GetSchemaResult>
function getSchemaOutput(args: GetSchemaOutputArgs, opts?: InvokeOptions): Output<GetSchemaResult>
def get_schema(database_id: Optional[str] = None,
id: Optional[str] = None,
name: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetSchemaResult
def get_schema_output(database_id: Optional[pulumi.Input[str]] = None,
id: Optional[pulumi.Input[str]] = None,
name: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetSchemaResult]
func LookupSchema(ctx *Context, args *LookupSchemaArgs, opts ...InvokeOption) (*LookupSchemaResult, error)
func LookupSchemaOutput(ctx *Context, args *LookupSchemaOutputArgs, opts ...InvokeOption) LookupSchemaResultOutput
> Note: This function is named LookupSchema
in the Go SDK.
public static class GetSchema
{
public static Task<GetSchemaResult> InvokeAsync(GetSchemaArgs args, InvokeOptions? opts = null)
public static Output<GetSchemaResult> Invoke(GetSchemaInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetSchemaResult> getSchema(GetSchemaArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: mssql:index/getSchema:getSchema
arguments:
# arguments dictionary
The following arguments are supported:
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Id string
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- Name string
- Schema name. Either
id
orname
must be provided.
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Id string
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- Name string
- Schema name. Either
id
orname
must be provided.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id String
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- name String
- Schema name. Either
id
orname
must be provided.
- database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id string
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- name string
- Schema name. Either
id
orname
must be provided.
- database_
id str - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id str
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- name str
- Schema name. Either
id
orname
must be provided.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id String
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- name String
- Schema name. Either
id
orname
must be provided.
getSchema Result
The following output properties are available:
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Id string
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- Name string
- Schema name. Either
id
orname
must be provided. - Owner
Id string - ID of database role or user owning this schema. Can be retrieved using
mssql.DatabaseRole
,mssql.SqlUser
,mssql.AzureadUser
ormssql.AzureadServicePrincipal
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Id string
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- Name string
- Schema name. Either
id
orname
must be provided. - Owner
Id string - ID of database role or user owning this schema. Can be retrieved using
mssql.DatabaseRole
,mssql.SqlUser
,mssql.AzureadUser
ormssql.AzureadServicePrincipal
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id String
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- name String
- Schema name. Either
id
orname
must be provided. - owner
Id String - ID of database role or user owning this schema. Can be retrieved using
mssql.DatabaseRole
,mssql.SqlUser
,mssql.AzureadUser
ormssql.AzureadServicePrincipal
- database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id string
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- name string
- Schema name. Either
id
orname
must be provided. - owner
Id string - ID of database role or user owning this schema. Can be retrieved using
mssql.DatabaseRole
,mssql.SqlUser
,mssql.AzureadUser
ormssql.AzureadServicePrincipal
- database_
id str - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id str
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- name str
- Schema name. Either
id
orname
must be provided. - owner_
id str - ID of database role or user owning this schema. Can be retrieved using
mssql.DatabaseRole
,mssql.SqlUser
,mssql.AzureadUser
ormssql.AzureadServicePrincipal
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id String
<database_id>/<schema_id>
. Schema ID can be retrieved usingSELECT SCHEMA_ID('<schema_name>')
. Eitherid
orname
must be provided.- name String
- Schema name. Either
id
orname
must be provided. - owner
Id String - ID of database role or user owning this schema. Can be retrieved using
mssql.DatabaseRole
,mssql.SqlUser
,mssql.AzureadUser
ormssql.AzureadServicePrincipal
Package Details
- Repository
- mssql pulumiverse/pulumi-mssql
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
mssql
Terraform Provider.
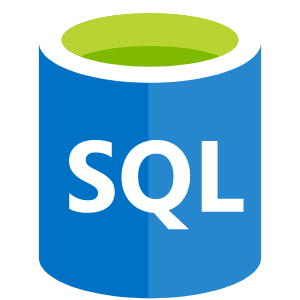
Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse