datarobot.CustomMetricFromJob
Explore with Pulumi AI
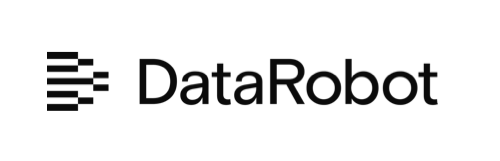
Custom Metric From Job
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const example = new datarobot.CustomMetricFromJob("example", {
deploymentId: datarobot_deployment.example.id,
customJobId: datarobot_custom_metric_job.example.id,
});
export const exampleId = example.id;
import pulumi
import pulumi_datarobot as datarobot
example = datarobot.CustomMetricFromJob("example",
deployment_id=datarobot_deployment["example"]["id"],
custom_job_id=datarobot_custom_metric_job["example"]["id"])
pulumi.export("exampleId", example.id)
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
example, err := datarobot.NewCustomMetricFromJob(ctx, "example", &datarobot.CustomMetricFromJobArgs{
DeploymentId: pulumi.Any(datarobot_deployment.Example.Id),
CustomJobId: pulumi.Any(datarobot_custom_metric_job.Example.Id),
})
if err != nil {
return err
}
ctx.Export("exampleId", example.ID())
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var example = new Datarobot.CustomMetricFromJob("example", new()
{
DeploymentId = datarobot_deployment.Example.Id,
CustomJobId = datarobot_custom_metric_job.Example.Id,
});
return new Dictionary<string, object?>
{
["exampleId"] = example.Id,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.CustomMetricFromJob;
import com.pulumi.datarobot.CustomMetricFromJobArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var example = new CustomMetricFromJob("example", CustomMetricFromJobArgs.builder()
.deploymentId(datarobot_deployment.example().id())
.customJobId(datarobot_custom_metric_job.example().id())
.build());
ctx.export("exampleId", example.id());
}
}
resources:
example:
type: datarobot:CustomMetricFromJob
properties:
deploymentId: ${datarobot_deployment.example.id}
customJobId: ${datarobot_custom_metric_job.example.id}
outputs:
exampleId: ${example.id}
Create CustomMetricFromJob Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new CustomMetricFromJob(name: string, args: CustomMetricFromJobArgs, opts?: CustomResourceOptions);
@overload
def CustomMetricFromJob(resource_name: str,
args: CustomMetricFromJobArgs,
opts: Optional[ResourceOptions] = None)
@overload
def CustomMetricFromJob(resource_name: str,
opts: Optional[ResourceOptions] = None,
custom_job_id: Optional[str] = None,
deployment_id: Optional[str] = None,
baseline_value: Optional[float] = None,
batch: Optional[CustomMetricFromJobBatchArgs] = None,
description: Optional[str] = None,
name: Optional[str] = None,
parameter_overrides: Optional[Sequence[CustomMetricFromJobParameterOverrideArgs]] = None,
sample_count: Optional[CustomMetricFromJobSampleCountArgs] = None,
schedule: Optional[CustomMetricFromJobScheduleArgs] = None,
timestamp: Optional[CustomMetricFromJobTimestampArgs] = None,
value: Optional[CustomMetricFromJobValueArgs] = None)
func NewCustomMetricFromJob(ctx *Context, name string, args CustomMetricFromJobArgs, opts ...ResourceOption) (*CustomMetricFromJob, error)
public CustomMetricFromJob(string name, CustomMetricFromJobArgs args, CustomResourceOptions? opts = null)
public CustomMetricFromJob(String name, CustomMetricFromJobArgs args)
public CustomMetricFromJob(String name, CustomMetricFromJobArgs args, CustomResourceOptions options)
type: datarobot:CustomMetricFromJob
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args CustomMetricFromJobArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args CustomMetricFromJobArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args CustomMetricFromJobArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args CustomMetricFromJobArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args CustomMetricFromJobArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var customMetricFromJobResource = new Datarobot.CustomMetricFromJob("customMetricFromJobResource", new()
{
CustomJobId = "string",
DeploymentId = "string",
BaselineValue = 0,
Batch = new Datarobot.Inputs.CustomMetricFromJobBatchArgs
{
ColumnName = "string",
},
Description = "string",
Name = "string",
ParameterOverrides = new[]
{
new Datarobot.Inputs.CustomMetricFromJobParameterOverrideArgs
{
Key = "string",
Type = "string",
Value = "string",
},
},
SampleCount = new Datarobot.Inputs.CustomMetricFromJobSampleCountArgs
{
ColumnName = "string",
},
Schedule = new Datarobot.Inputs.CustomMetricFromJobScheduleArgs
{
DayOfMonths = new[]
{
"string",
},
DayOfWeeks = new[]
{
"string",
},
Hours = new[]
{
"string",
},
Minutes = new[]
{
"string",
},
Months = new[]
{
"string",
},
},
Timestamp = new Datarobot.Inputs.CustomMetricFromJobTimestampArgs
{
ColumnName = "string",
TimeFormat = "string",
},
Value = new Datarobot.Inputs.CustomMetricFromJobValueArgs
{
ColumnName = "string",
},
});
example, err := datarobot.NewCustomMetricFromJob(ctx, "customMetricFromJobResource", &datarobot.CustomMetricFromJobArgs{
CustomJobId: pulumi.String("string"),
DeploymentId: pulumi.String("string"),
BaselineValue: pulumi.Float64(0),
Batch: &datarobot.CustomMetricFromJobBatchArgs{
ColumnName: pulumi.String("string"),
},
Description: pulumi.String("string"),
Name: pulumi.String("string"),
ParameterOverrides: datarobot.CustomMetricFromJobParameterOverrideArray{
&datarobot.CustomMetricFromJobParameterOverrideArgs{
Key: pulumi.String("string"),
Type: pulumi.String("string"),
Value: pulumi.String("string"),
},
},
SampleCount: &datarobot.CustomMetricFromJobSampleCountArgs{
ColumnName: pulumi.String("string"),
},
Schedule: &datarobot.CustomMetricFromJobScheduleArgs{
DayOfMonths: pulumi.StringArray{
pulumi.String("string"),
},
DayOfWeeks: pulumi.StringArray{
pulumi.String("string"),
},
Hours: pulumi.StringArray{
pulumi.String("string"),
},
Minutes: pulumi.StringArray{
pulumi.String("string"),
},
Months: pulumi.StringArray{
pulumi.String("string"),
},
},
Timestamp: &datarobot.CustomMetricFromJobTimestampArgs{
ColumnName: pulumi.String("string"),
TimeFormat: pulumi.String("string"),
},
Value: &datarobot.CustomMetricFromJobValueArgs{
ColumnName: pulumi.String("string"),
},
})
var customMetricFromJobResource = new CustomMetricFromJob("customMetricFromJobResource", CustomMetricFromJobArgs.builder()
.customJobId("string")
.deploymentId("string")
.baselineValue(0.0)
.batch(CustomMetricFromJobBatchArgs.builder()
.columnName("string")
.build())
.description("string")
.name("string")
.parameterOverrides(CustomMetricFromJobParameterOverrideArgs.builder()
.key("string")
.type("string")
.value("string")
.build())
.sampleCount(CustomMetricFromJobSampleCountArgs.builder()
.columnName("string")
.build())
.schedule(CustomMetricFromJobScheduleArgs.builder()
.dayOfMonths("string")
.dayOfWeeks("string")
.hours("string")
.minutes("string")
.months("string")
.build())
.timestamp(CustomMetricFromJobTimestampArgs.builder()
.columnName("string")
.timeFormat("string")
.build())
.value(CustomMetricFromJobValueArgs.builder()
.columnName("string")
.build())
.build());
custom_metric_from_job_resource = datarobot.CustomMetricFromJob("customMetricFromJobResource",
custom_job_id="string",
deployment_id="string",
baseline_value=0,
batch={
"column_name": "string",
},
description="string",
name="string",
parameter_overrides=[{
"key": "string",
"type": "string",
"value": "string",
}],
sample_count={
"column_name": "string",
},
schedule={
"day_of_months": ["string"],
"day_of_weeks": ["string"],
"hours": ["string"],
"minutes": ["string"],
"months": ["string"],
},
timestamp={
"column_name": "string",
"time_format": "string",
},
value={
"column_name": "string",
})
const customMetricFromJobResource = new datarobot.CustomMetricFromJob("customMetricFromJobResource", {
customJobId: "string",
deploymentId: "string",
baselineValue: 0,
batch: {
columnName: "string",
},
description: "string",
name: "string",
parameterOverrides: [{
key: "string",
type: "string",
value: "string",
}],
sampleCount: {
columnName: "string",
},
schedule: {
dayOfMonths: ["string"],
dayOfWeeks: ["string"],
hours: ["string"],
minutes: ["string"],
months: ["string"],
},
timestamp: {
columnName: "string",
timeFormat: "string",
},
value: {
columnName: "string",
},
});
type: datarobot:CustomMetricFromJob
properties:
baselineValue: 0
batch:
columnName: string
customJobId: string
deploymentId: string
description: string
name: string
parameterOverrides:
- key: string
type: string
value: string
sampleCount:
columnName: string
schedule:
dayOfMonths:
- string
dayOfWeeks:
- string
hours:
- string
minutes:
- string
months:
- string
timestamp:
columnName: string
timeFormat: string
value:
columnName: string
CustomMetricFromJob Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The CustomMetricFromJob resource accepts the following input properties:
- Custom
Job stringId - ID of the Custom Job.
- Deployment
Id string - ID of the Deployment.
- Baseline
Value double - Baseline value for the metric.
- Batch
Data
Robot Custom Metric From Job Batch - Batch ID source when reading values from columnar dataset like a file.
- Description string
- Description of the metric.
- Name string
- Name of the metric.
- Parameter
Overrides List<DataRobot Custom Metric From Job Parameter Override> - Additional parameters to be injected into the Metric Job at runtime.
- Sample
Count DataRobot Custom Metric From Job Sample Count - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- Schedule
Data
Robot Custom Metric From Job Schedule - Defines at what intervals the metric job should run.
- Timestamp
Data
Robot Custom Metric From Job Timestamp - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- Value
Data
Robot Custom Metric From Job Value - Value source when reading values from columnar dataset like a file.
- Custom
Job stringId - ID of the Custom Job.
- Deployment
Id string - ID of the Deployment.
- Baseline
Value float64 - Baseline value for the metric.
- Batch
Custom
Metric From Job Batch Args - Batch ID source when reading values from columnar dataset like a file.
- Description string
- Description of the metric.
- Name string
- Name of the metric.
- Parameter
Overrides []CustomMetric From Job Parameter Override Args - Additional parameters to be injected into the Metric Job at runtime.
- Sample
Count CustomMetric From Job Sample Count Args - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- Schedule
Custom
Metric From Job Schedule Args - Defines at what intervals the metric job should run.
- Timestamp
Custom
Metric From Job Timestamp Args - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- Value
Custom
Metric From Job Value Args - Value source when reading values from columnar dataset like a file.
- custom
Job StringId - ID of the Custom Job.
- deployment
Id String - ID of the Deployment.
- baseline
Value Double - Baseline value for the metric.
- batch
Custom
Metric From Job Batch - Batch ID source when reading values from columnar dataset like a file.
- description String
- Description of the metric.
- name String
- Name of the metric.
- parameter
Overrides List<CustomMetric From Job Parameter Override> - Additional parameters to be injected into the Metric Job at runtime.
- sample
Count CustomMetric From Job Sample Count - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- schedule
Custom
Metric From Job Schedule - Defines at what intervals the metric job should run.
- timestamp
Custom
Metric From Job Timestamp - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- value
Custom
Metric From Job Value - Value source when reading values from columnar dataset like a file.
- custom
Job stringId - ID of the Custom Job.
- deployment
Id string - ID of the Deployment.
- baseline
Value number - Baseline value for the metric.
- batch
Custom
Metric From Job Batch - Batch ID source when reading values from columnar dataset like a file.
- description string
- Description of the metric.
- name string
- Name of the metric.
- parameter
Overrides CustomMetric From Job Parameter Override[] - Additional parameters to be injected into the Metric Job at runtime.
- sample
Count CustomMetric From Job Sample Count - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- schedule
Custom
Metric From Job Schedule - Defines at what intervals the metric job should run.
- timestamp
Custom
Metric From Job Timestamp - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- value
Custom
Metric From Job Value - Value source when reading values from columnar dataset like a file.
- custom_
job_ strid - ID of the Custom Job.
- deployment_
id str - ID of the Deployment.
- baseline_
value float - Baseline value for the metric.
- batch
Custom
Metric From Job Batch Args - Batch ID source when reading values from columnar dataset like a file.
- description str
- Description of the metric.
- name str
- Name of the metric.
- parameter_
overrides Sequence[CustomMetric From Job Parameter Override Args] - Additional parameters to be injected into the Metric Job at runtime.
- sample_
count CustomMetric From Job Sample Count Args - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- schedule
Custom
Metric From Job Schedule Args - Defines at what intervals the metric job should run.
- timestamp
Custom
Metric From Job Timestamp Args - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- value
Custom
Metric From Job Value Args - Value source when reading values from columnar dataset like a file.
- custom
Job StringId - ID of the Custom Job.
- deployment
Id String - ID of the Deployment.
- baseline
Value Number - Baseline value for the metric.
- batch Property Map
- Batch ID source when reading values from columnar dataset like a file.
- description String
- Description of the metric.
- name String
- Name of the metric.
- parameter
Overrides List<Property Map> - Additional parameters to be injected into the Metric Job at runtime.
- sample
Count Property Map - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- schedule Property Map
- Defines at what intervals the metric job should run.
- timestamp Property Map
- Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- value Property Map
- Value source when reading values from columnar dataset like a file.
Outputs
All input properties are implicitly available as output properties. Additionally, the CustomMetricFromJob resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing CustomMetricFromJob Resource
Get an existing CustomMetricFromJob resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: CustomMetricFromJobState, opts?: CustomResourceOptions): CustomMetricFromJob
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
baseline_value: Optional[float] = None,
batch: Optional[CustomMetricFromJobBatchArgs] = None,
custom_job_id: Optional[str] = None,
deployment_id: Optional[str] = None,
description: Optional[str] = None,
name: Optional[str] = None,
parameter_overrides: Optional[Sequence[CustomMetricFromJobParameterOverrideArgs]] = None,
sample_count: Optional[CustomMetricFromJobSampleCountArgs] = None,
schedule: Optional[CustomMetricFromJobScheduleArgs] = None,
timestamp: Optional[CustomMetricFromJobTimestampArgs] = None,
value: Optional[CustomMetricFromJobValueArgs] = None) -> CustomMetricFromJob
func GetCustomMetricFromJob(ctx *Context, name string, id IDInput, state *CustomMetricFromJobState, opts ...ResourceOption) (*CustomMetricFromJob, error)
public static CustomMetricFromJob Get(string name, Input<string> id, CustomMetricFromJobState? state, CustomResourceOptions? opts = null)
public static CustomMetricFromJob get(String name, Output<String> id, CustomMetricFromJobState state, CustomResourceOptions options)
resources: _: type: datarobot:CustomMetricFromJob get: id: ${id}
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Baseline
Value double - Baseline value for the metric.
- Batch
Data
Robot Custom Metric From Job Batch - Batch ID source when reading values from columnar dataset like a file.
- Custom
Job stringId - ID of the Custom Job.
- Deployment
Id string - ID of the Deployment.
- Description string
- Description of the metric.
- Name string
- Name of the metric.
- Parameter
Overrides List<DataRobot Custom Metric From Job Parameter Override> - Additional parameters to be injected into the Metric Job at runtime.
- Sample
Count DataRobot Custom Metric From Job Sample Count - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- Schedule
Data
Robot Custom Metric From Job Schedule - Defines at what intervals the metric job should run.
- Timestamp
Data
Robot Custom Metric From Job Timestamp - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- Value
Data
Robot Custom Metric From Job Value - Value source when reading values from columnar dataset like a file.
- Baseline
Value float64 - Baseline value for the metric.
- Batch
Custom
Metric From Job Batch Args - Batch ID source when reading values from columnar dataset like a file.
- Custom
Job stringId - ID of the Custom Job.
- Deployment
Id string - ID of the Deployment.
- Description string
- Description of the metric.
- Name string
- Name of the metric.
- Parameter
Overrides []CustomMetric From Job Parameter Override Args - Additional parameters to be injected into the Metric Job at runtime.
- Sample
Count CustomMetric From Job Sample Count Args - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- Schedule
Custom
Metric From Job Schedule Args - Defines at what intervals the metric job should run.
- Timestamp
Custom
Metric From Job Timestamp Args - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- Value
Custom
Metric From Job Value Args - Value source when reading values from columnar dataset like a file.
- baseline
Value Double - Baseline value for the metric.
- batch
Custom
Metric From Job Batch - Batch ID source when reading values from columnar dataset like a file.
- custom
Job StringId - ID of the Custom Job.
- deployment
Id String - ID of the Deployment.
- description String
- Description of the metric.
- name String
- Name of the metric.
- parameter
Overrides List<CustomMetric From Job Parameter Override> - Additional parameters to be injected into the Metric Job at runtime.
- sample
Count CustomMetric From Job Sample Count - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- schedule
Custom
Metric From Job Schedule - Defines at what intervals the metric job should run.
- timestamp
Custom
Metric From Job Timestamp - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- value
Custom
Metric From Job Value - Value source when reading values from columnar dataset like a file.
- baseline
Value number - Baseline value for the metric.
- batch
Custom
Metric From Job Batch - Batch ID source when reading values from columnar dataset like a file.
- custom
Job stringId - ID of the Custom Job.
- deployment
Id string - ID of the Deployment.
- description string
- Description of the metric.
- name string
- Name of the metric.
- parameter
Overrides CustomMetric From Job Parameter Override[] - Additional parameters to be injected into the Metric Job at runtime.
- sample
Count CustomMetric From Job Sample Count - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- schedule
Custom
Metric From Job Schedule - Defines at what intervals the metric job should run.
- timestamp
Custom
Metric From Job Timestamp - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- value
Custom
Metric From Job Value - Value source when reading values from columnar dataset like a file.
- baseline_
value float - Baseline value for the metric.
- batch
Custom
Metric From Job Batch Args - Batch ID source when reading values from columnar dataset like a file.
- custom_
job_ strid - ID of the Custom Job.
- deployment_
id str - ID of the Deployment.
- description str
- Description of the metric.
- name str
- Name of the metric.
- parameter_
overrides Sequence[CustomMetric From Job Parameter Override Args] - Additional parameters to be injected into the Metric Job at runtime.
- sample_
count CustomMetric From Job Sample Count Args - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- schedule
Custom
Metric From Job Schedule Args - Defines at what intervals the metric job should run.
- timestamp
Custom
Metric From Job Timestamp Args - Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- value
Custom
Metric From Job Value Args - Value source when reading values from columnar dataset like a file.
- baseline
Value Number - Baseline value for the metric.
- batch Property Map
- Batch ID source when reading values from columnar dataset like a file.
- custom
Job StringId - ID of the Custom Job.
- deployment
Id String - ID of the Deployment.
- description String
- Description of the metric.
- name String
- Name of the metric.
- parameter
Overrides List<Property Map> - Additional parameters to be injected into the Metric Job at runtime.
- sample
Count Property Map - Points to a weight column if users provide pre-aggregated metric values. Used with columnar datasets.
- schedule Property Map
- Defines at what intervals the metric job should run.
- timestamp Property Map
- Timestamp spoofing when reading values from file, like dataset. By default, we replicate pd.to_datetime formatting behaviour.
- value Property Map
- Value source when reading values from columnar dataset like a file.
Supporting Types
CustomMetricFromJobBatch, CustomMetricFromJobBatchArgs
- Column
Name string - Column name.
- Column
Name string - Column name.
- column
Name String - Column name.
- column
Name string - Column name.
- column_
name str - Column name.
- column
Name String - Column name.
CustomMetricFromJobParameterOverride, CustomMetricFromJobParameterOverrideArgs
CustomMetricFromJobSampleCount, CustomMetricFromJobSampleCountArgs
- Column
Name string - Column name.
- Column
Name string - Column name.
- column
Name String - Column name.
- column
Name string - Column name.
- column_
name str - Column name.
- column
Name String - Column name.
CustomMetricFromJobSchedule, CustomMetricFromJobScheduleArgs
- Day
Of List<string>Months - Days of the month when the metric job will run.
- Day
Of List<string>Weeks - Days of the week when the metric job will run.
- Hours List<string>
- Hours of the day when the metric job will run.
- Minutes List<string>
- Minutes of the day when the metric job will run.
- Months List<string>
- Months of the year when the metric job will run.
- Day
Of []stringMonths - Days of the month when the metric job will run.
- Day
Of []stringWeeks - Days of the week when the metric job will run.
- Hours []string
- Hours of the day when the metric job will run.
- Minutes []string
- Minutes of the day when the metric job will run.
- Months []string
- Months of the year when the metric job will run.
- day
Of List<String>Months - Days of the month when the metric job will run.
- day
Of List<String>Weeks - Days of the week when the metric job will run.
- hours List<String>
- Hours of the day when the metric job will run.
- minutes List<String>
- Minutes of the day when the metric job will run.
- months List<String>
- Months of the year when the metric job will run.
- day
Of string[]Months - Days of the month when the metric job will run.
- day
Of string[]Weeks - Days of the week when the metric job will run.
- hours string[]
- Hours of the day when the metric job will run.
- minutes string[]
- Minutes of the day when the metric job will run.
- months string[]
- Months of the year when the metric job will run.
- day_
of_ Sequence[str]months - Days of the month when the metric job will run.
- day_
of_ Sequence[str]weeks - Days of the week when the metric job will run.
- hours Sequence[str]
- Hours of the day when the metric job will run.
- minutes Sequence[str]
- Minutes of the day when the metric job will run.
- months Sequence[str]
- Months of the year when the metric job will run.
- day
Of List<String>Months - Days of the month when the metric job will run.
- day
Of List<String>Weeks - Days of the week when the metric job will run.
- hours List<String>
- Hours of the day when the metric job will run.
- minutes List<String>
- Minutes of the day when the metric job will run.
- months List<String>
- Months of the year when the metric job will run.
CustomMetricFromJobTimestamp, CustomMetricFromJobTimestampArgs
- Column
Name string - Column name.
- Time
Format string - Format.
- Column
Name string - Column name.
- Time
Format string - Format.
- column
Name String - Column name.
- time
Format String - Format.
- column
Name string - Column name.
- time
Format string - Format.
- column_
name str - Column name.
- time_
format str - Format.
- column
Name String - Column name.
- time
Format String - Format.
CustomMetricFromJobValue, CustomMetricFromJobValueArgs
- Column
Name string - Column name.
- Column
Name string - Column name.
- column
Name String - Column name.
- column
Name string - Column name.
- column_
name str - Column name.
- column
Name String - Column name.
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
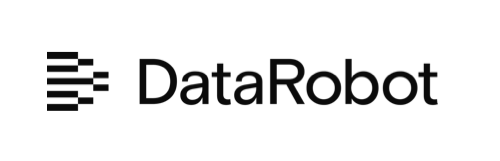