Proxmox Virtual Environment (Proxmox VE) v6.7.0 published on Saturday, May 18, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.HA.getHAGroup
Explore with Pulumi AI
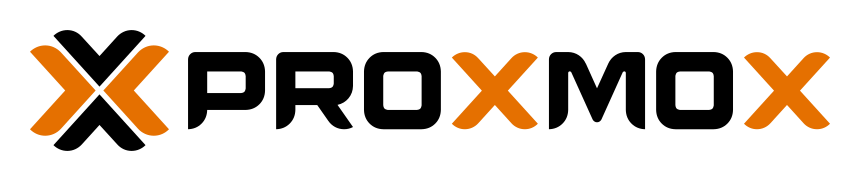
Proxmox Virtual Environment (Proxmox VE) v6.7.0 published on Saturday, May 18, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves information about a specific High Availability group.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const all = proxmoxve.HA.getHAGroups({});
const example = all.then(all => .map(([, ]) => (proxmoxve.HA.getHAGroup({
group: __value,
}))));
export const proxmoxVirtualEnvironmentHagroupsFull = example;
import pulumi
import pulumi_proxmoxve as proxmoxve
all = proxmoxve.HA.get_ha_groups()
example = [proxmoxve.HA.get_ha_group(group=__value) for __key, __value in all.group_ids]
pulumi.export("proxmoxVirtualEnvironmentHagroupsFull", example)
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/HA"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
all, err := HA.GetHAGroups(ctx, nil, nil)
if err != nil {
return err
}
example := "TODO: For expression"
ctx.Export("proxmoxVirtualEnvironmentHagroupsFull", example)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var all = ProxmoxVE.HA.GetHAGroups.Invoke();
var example = .Select(__value =>
{
return ProxmoxVE.HA.GetHAGroup.Invoke(new()
{
Group = __value,
});
}).ToList();
return new Dictionary<string, object?>
{
["proxmoxVirtualEnvironmentHagroupsFull"] = example,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.HA.HAFunctions;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var all = HAFunctions.getHAGroups();
final var example = "TODO: ForExpression";
ctx.export("proxmoxVirtualEnvironmentHagroupsFull", example);
}
}
Coming soon!
Using getHAGroup
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getHAGroup(args: GetHAGroupArgs, opts?: InvokeOptions): Promise<GetHAGroupResult>
function getHAGroupOutput(args: GetHAGroupOutputArgs, opts?: InvokeOptions): Output<GetHAGroupResult>
def get_ha_group(group: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetHAGroupResult
def get_ha_group_output(group: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetHAGroupResult]
func GetHAGroup(ctx *Context, args *GetHAGroupArgs, opts ...InvokeOption) (*GetHAGroupResult, error)
func GetHAGroupOutput(ctx *Context, args *GetHAGroupOutputArgs, opts ...InvokeOption) GetHAGroupResultOutput
> Note: This function is named GetHAGroup
in the Go SDK.
public static class GetHAGroup
{
public static Task<GetHAGroupResult> InvokeAsync(GetHAGroupArgs args, InvokeOptions? opts = null)
public static Output<GetHAGroupResult> Invoke(GetHAGroupInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetHAGroupResult> getHAGroup(GetHAGroupArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:HA/getHAGroup:getHAGroup
arguments:
# arguments dictionary
The following arguments are supported:
- Group string
- The identifier of the High Availability group to read.
- Group string
- The identifier of the High Availability group to read.
- group String
- The identifier of the High Availability group to read.
- group string
- The identifier of the High Availability group to read.
- group str
- The identifier of the High Availability group to read.
- group String
- The identifier of the High Availability group to read.
getHAGroup Result
The following output properties are available:
- Comment string
- The comment associated with this group
- Group string
- The identifier of the High Availability group to read.
- Id string
- The unique identifier of this resource.
- No
Failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group.
- Nodes Dictionary<string, int>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - Restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group.
- Comment string
- The comment associated with this group
- Group string
- The identifier of the High Availability group to read.
- Id string
- The unique identifier of this resource.
- No
Failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group.
- Nodes map[string]int
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - Restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group.
- comment String
- The comment associated with this group
- group String
- The identifier of the High Availability group to read.
- id String
- The unique identifier of this resource.
- no
Failback Boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group.
- nodes Map<String,Integer>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - restricted Boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group.
- comment string
- The comment associated with this group
- group string
- The identifier of the High Availability group to read.
- id string
- The unique identifier of this resource.
- no
Failback boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group.
- nodes {[key: string]: number}
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - restricted boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group.
- comment str
- The comment associated with this group
- group str
- The identifier of the High Availability group to read.
- id str
- The unique identifier of this resource.
- no_
failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group.
- nodes Mapping[str, int]
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group.
- comment String
- The comment associated with this group
- group String
- The identifier of the High Availability group to read.
- id String
- The unique identifier of this resource.
- no
Failback Boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group.
- nodes Map<Number>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - restricted Boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
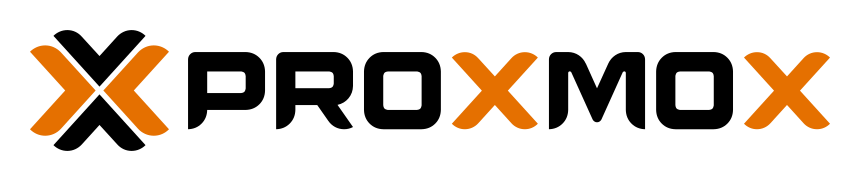
Proxmox Virtual Environment (Proxmox VE) v6.7.0 published on Saturday, May 18, 2024 by Daniel Muehlbachler-Pietrzykowski