Proxmox Virtual Environment (Proxmox VE) v6.7.0 published on Saturday, May 18, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.HA.getHAResource
Explore with Pulumi AI
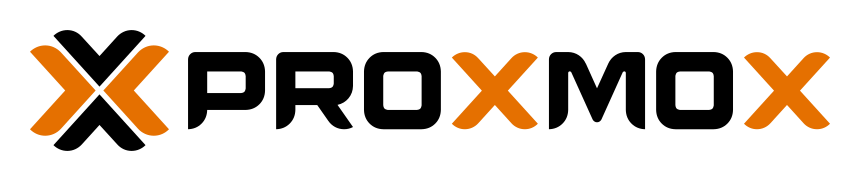
Proxmox Virtual Environment (Proxmox VE) v6.7.0 published on Saturday, May 18, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves the list of High Availability resources.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const all = proxmoxve.HA.getHAResources({});
const example = all.then(all => .map(([, ]) => (proxmoxve.HA.getHAResource({
resourceId: __value,
}))));
export const proxmoxVirtualEnvironmentHaresourcesFull = example;
import pulumi
import pulumi_proxmoxve as proxmoxve
all = proxmoxve.HA.get_ha_resources()
example = [proxmoxve.HA.get_ha_resource(resource_id=__value) for __key, __value in all.resource_ids]
pulumi.export("proxmoxVirtualEnvironmentHaresourcesFull", example)
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/HA"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
all, err := HA.GetHAResources(ctx, nil, nil)
if err != nil {
return err
}
example := "TODO: For expression"
ctx.Export("proxmoxVirtualEnvironmentHaresourcesFull", example)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var all = ProxmoxVE.HA.GetHAResources.Invoke();
var example = .Select(__value =>
{
return ProxmoxVE.HA.GetHAResource.Invoke(new()
{
ResourceId = __value,
});
}).ToList();
return new Dictionary<string, object?>
{
["proxmoxVirtualEnvironmentHaresourcesFull"] = example,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.HA.HAFunctions;
import com.pulumi.proxmoxve.HA.inputs.GetHAResourcesArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var all = HAFunctions.getHAResources();
final var example = "TODO: ForExpression";
ctx.export("proxmoxVirtualEnvironmentHaresourcesFull", example);
}
}
Coming soon!
Using getHAResource
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getHAResource(args: GetHAResourceArgs, opts?: InvokeOptions): Promise<GetHAResourceResult>
function getHAResourceOutput(args: GetHAResourceOutputArgs, opts?: InvokeOptions): Output<GetHAResourceResult>
def get_ha_resource(resource_id: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetHAResourceResult
def get_ha_resource_output(resource_id: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetHAResourceResult]
func GetHAResource(ctx *Context, args *GetHAResourceArgs, opts ...InvokeOption) (*GetHAResourceResult, error)
func GetHAResourceOutput(ctx *Context, args *GetHAResourceOutputArgs, opts ...InvokeOption) GetHAResourceResultOutput
> Note: This function is named GetHAResource
in the Go SDK.
public static class GetHAResource
{
public static Task<GetHAResourceResult> InvokeAsync(GetHAResourceArgs args, InvokeOptions? opts = null)
public static Output<GetHAResourceResult> Invoke(GetHAResourceInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetHAResourceResult> getHAResource(GetHAResourceArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:HA/getHAResource:getHAResource
arguments:
# arguments dictionary
The following arguments are supported:
- Resource
Id string - The identifier of the Proxmox HA resource to read.
- Resource
Id string - The identifier of the Proxmox HA resource to read.
- resource
Id String - The identifier of the Proxmox HA resource to read.
- resource
Id string - The identifier of the Proxmox HA resource to read.
- resource_
id str - The identifier of the Proxmox HA resource to read.
- resource
Id String - The identifier of the Proxmox HA resource to read.
getHAResource Result
The following output properties are available:
- Comment string
- The comment associated with this resource.
- Group string
- The identifier of the High Availability group this resource is a member of.
- Id string
- The unique identifier of this resource.
- Max
Relocate int - The maximal number of relocation attempts.
- Max
Restart int - The maximal number of restart attempts.
- Resource
Id string - The identifier of the Proxmox HA resource to read.
- State string
- The desired state of the resource.
- Type string
- The type of High Availability resource (
vm
orct
).
- Comment string
- The comment associated with this resource.
- Group string
- The identifier of the High Availability group this resource is a member of.
- Id string
- The unique identifier of this resource.
- Max
Relocate int - The maximal number of relocation attempts.
- Max
Restart int - The maximal number of restart attempts.
- Resource
Id string - The identifier of the Proxmox HA resource to read.
- State string
- The desired state of the resource.
- Type string
- The type of High Availability resource (
vm
orct
).
- comment String
- The comment associated with this resource.
- group String
- The identifier of the High Availability group this resource is a member of.
- id String
- The unique identifier of this resource.
- max
Relocate Integer - The maximal number of relocation attempts.
- max
Restart Integer - The maximal number of restart attempts.
- resource
Id String - The identifier of the Proxmox HA resource to read.
- state String
- The desired state of the resource.
- type String
- The type of High Availability resource (
vm
orct
).
- comment string
- The comment associated with this resource.
- group string
- The identifier of the High Availability group this resource is a member of.
- id string
- The unique identifier of this resource.
- max
Relocate number - The maximal number of relocation attempts.
- max
Restart number - The maximal number of restart attempts.
- resource
Id string - The identifier of the Proxmox HA resource to read.
- state string
- The desired state of the resource.
- type string
- The type of High Availability resource (
vm
orct
).
- comment str
- The comment associated with this resource.
- group str
- The identifier of the High Availability group this resource is a member of.
- id str
- The unique identifier of this resource.
- max_
relocate int - The maximal number of relocation attempts.
- max_
restart int - The maximal number of restart attempts.
- resource_
id str - The identifier of the Proxmox HA resource to read.
- state str
- The desired state of the resource.
- type str
- The type of High Availability resource (
vm
orct
).
- comment String
- The comment associated with this resource.
- group String
- The identifier of the High Availability group this resource is a member of.
- id String
- The unique identifier of this resource.
- max
Relocate Number - The maximal number of relocation attempts.
- max
Restart Number - The maximal number of restart attempts.
- resource
Id String - The identifier of the Proxmox HA resource to read.
- state String
- The desired state of the resource.
- type String
- The type of High Availability resource (
vm
orct
).
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
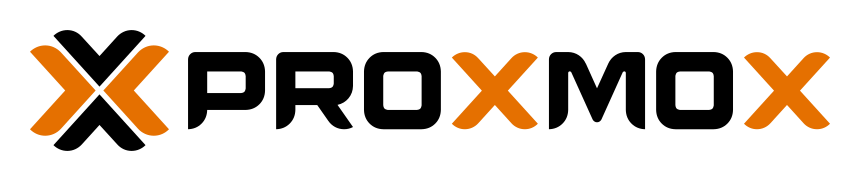
Proxmox Virtual Environment (Proxmox VE) v6.7.0 published on Saturday, May 18, 2024 by Daniel Muehlbachler-Pietrzykowski