Proxmox Virtual Environment (Proxmox VE) v7.2.0 published on Sunday, Jun 29, 2025 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Hardware.getMappings
Explore with Pulumi AI
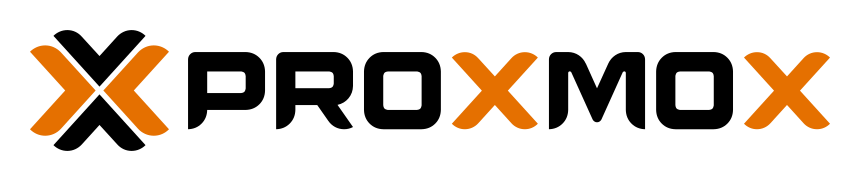
Proxmox Virtual Environment (Proxmox VE) v7.2.0 published on Sunday, Jun 29, 2025 by Daniel Muehlbachler-Pietrzykowski
Retrieves a list of hardware mapping resources.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const example_dir = proxmoxve.Hardware.getMappings({
checkNode: "pve",
type: "dir",
});
const example_pci = proxmoxve.Hardware.getMappings({
checkNode: "pve",
type: "pci",
});
const example_usb = proxmoxve.Hardware.getMappings({
checkNode: "pve",
type: "usb",
});
export const dataProxmoxVirtualEnvironmentHardwareMappingsPci = example_pci;
export const dataProxmoxVirtualEnvironmentHardwareMappingsUsb = example_usb;
import pulumi
import pulumi_proxmoxve as proxmoxve
example_dir = proxmoxve.Hardware.get_mappings(check_node="pve",
type="dir")
example_pci = proxmoxve.Hardware.get_mappings(check_node="pve",
type="pci")
example_usb = proxmoxve.Hardware.get_mappings(check_node="pve",
type="usb")
pulumi.export("dataProxmoxVirtualEnvironmentHardwareMappingsPci", example_pci)
pulumi.export("dataProxmoxVirtualEnvironmentHardwareMappingsUsb", example_usb)
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v7/go/proxmoxve/hardware"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := hardware.GetMappings(ctx, &hardware.GetMappingsArgs{
CheckNode: pulumi.StringRef("pve"),
Type: "dir",
}, nil)
if err != nil {
return err
}
example_pci, err := hardware.GetMappings(ctx, &hardware.GetMappingsArgs{
CheckNode: pulumi.StringRef("pve"),
Type: "pci",
}, nil)
if err != nil {
return err
}
example_usb, err := hardware.GetMappings(ctx, &hardware.GetMappingsArgs{
CheckNode: pulumi.StringRef("pve"),
Type: "usb",
}, nil)
if err != nil {
return err
}
ctx.Export("dataProxmoxVirtualEnvironmentHardwareMappingsPci", example_pci)
ctx.Export("dataProxmoxVirtualEnvironmentHardwareMappingsUsb", example_usb)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example_dir = ProxmoxVE.Hardware.GetMappings.Invoke(new()
{
CheckNode = "pve",
Type = "dir",
});
var example_pci = ProxmoxVE.Hardware.GetMappings.Invoke(new()
{
CheckNode = "pve",
Type = "pci",
});
var example_usb = ProxmoxVE.Hardware.GetMappings.Invoke(new()
{
CheckNode = "pve",
Type = "usb",
});
return new Dictionary<string, object?>
{
["dataProxmoxVirtualEnvironmentHardwareMappingsPci"] = example_pci,
["dataProxmoxVirtualEnvironmentHardwareMappingsUsb"] = example_usb,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Hardware.HardwareFunctions;
import com.pulumi.proxmoxve.Hardware.inputs.GetMappingsArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var example-dir = HardwareFunctions.getMappings(GetMappingsArgs.builder()
.checkNode("pve")
.type("dir")
.build());
final var example-pci = HardwareFunctions.getMappings(GetMappingsArgs.builder()
.checkNode("pve")
.type("pci")
.build());
final var example-usb = HardwareFunctions.getMappings(GetMappingsArgs.builder()
.checkNode("pve")
.type("usb")
.build());
ctx.export("dataProxmoxVirtualEnvironmentHardwareMappingsPci", example_pci);
ctx.export("dataProxmoxVirtualEnvironmentHardwareMappingsUsb", example_usb);
}
}
variables:
example-dir:
fn::invoke:
function: proxmoxve:Hardware:getMappings
arguments:
checkNode: pve
type: dir
example-pci:
fn::invoke:
function: proxmoxve:Hardware:getMappings
arguments:
checkNode: pve
type: pci
example-usb:
fn::invoke:
function: proxmoxve:Hardware:getMappings
arguments:
checkNode: pve
type: usb
outputs:
dataProxmoxVirtualEnvironmentHardwareMappingsPci: ${["example-pci"]}
dataProxmoxVirtualEnvironmentHardwareMappingsUsb: ${["example-usb"]}
Using getMappings
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getMappings(args: GetMappingsArgs, opts?: InvokeOptions): Promise<GetMappingsResult>
function getMappingsOutput(args: GetMappingsOutputArgs, opts?: InvokeOptions): Output<GetMappingsResult>
def get_mappings(check_node: Optional[str] = None,
type: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetMappingsResult
def get_mappings_output(check_node: Optional[pulumi.Input[str]] = None,
type: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetMappingsResult]
func GetMappings(ctx *Context, args *GetMappingsArgs, opts ...InvokeOption) (*GetMappingsResult, error)
func GetMappingsOutput(ctx *Context, args *GetMappingsOutputArgs, opts ...InvokeOption) GetMappingsResultOutput
> Note: This function is named GetMappings
in the Go SDK.
public static class GetMappings
{
public static Task<GetMappingsResult> InvokeAsync(GetMappingsArgs args, InvokeOptions? opts = null)
public static Output<GetMappingsResult> Invoke(GetMappingsInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetMappingsResult> getMappings(GetMappingsArgs args, InvokeOptions options)
public static Output<GetMappingsResult> getMappings(GetMappingsArgs args, InvokeOptions options)
fn::invoke:
function: proxmoxve:Hardware/getMappings:getMappings
arguments:
# arguments dictionary
The following arguments are supported:
- type str
- The type of the hardware mappings.
- check_
node str - The name of the node whose configurations should be checked for correctness.
getMappings Result
The following output properties are available:
- Checks
List<Pulumi.
Proxmox VE. Hardware. Outputs. Get Mappings Check> - Might contain relevant diagnostics about incorrect configurations.
- Id string
- The unique identifier of this hardware mappings data source.
- Ids List<string>
- The identifiers of the hardware mappings.
- Type string
- The type of the hardware mappings.
- Check
Node string - The name of the node whose configurations should be checked for correctness.
- Checks
[]Get
Mappings Check - Might contain relevant diagnostics about incorrect configurations.
- Id string
- The unique identifier of this hardware mappings data source.
- Ids []string
- The identifiers of the hardware mappings.
- Type string
- The type of the hardware mappings.
- Check
Node string - The name of the node whose configurations should be checked for correctness.
- checks
List<Get
Mappings Check> - Might contain relevant diagnostics about incorrect configurations.
- id String
- The unique identifier of this hardware mappings data source.
- ids List<String>
- The identifiers of the hardware mappings.
- type String
- The type of the hardware mappings.
- check
Node String - The name of the node whose configurations should be checked for correctness.
- checks
Get
Mappings Check[] - Might contain relevant diagnostics about incorrect configurations.
- id string
- The unique identifier of this hardware mappings data source.
- ids string[]
- The identifiers of the hardware mappings.
- type string
- The type of the hardware mappings.
- check
Node string - The name of the node whose configurations should be checked for correctness.
- checks
Sequence[Get
Mappings Check] - Might contain relevant diagnostics about incorrect configurations.
- id str
- The unique identifier of this hardware mappings data source.
- ids Sequence[str]
- The identifiers of the hardware mappings.
- type str
- The type of the hardware mappings.
- check_
node str - The name of the node whose configurations should be checked for correctness.
- checks List<Property Map>
- Might contain relevant diagnostics about incorrect configurations.
- id String
- The unique identifier of this hardware mappings data source.
- ids List<String>
- The identifiers of the hardware mappings.
- type String
- The type of the hardware mappings.
- check
Node String - The name of the node whose configurations should be checked for correctness.
Supporting Types
GetMappingsCheck
- mapping_
id str - The corresponding hardware mapping ID of the node check diagnostic entry.
- message str
- The message of the node check diagnostic entry.
- severity str
- The severity of the node check diagnostic entry.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
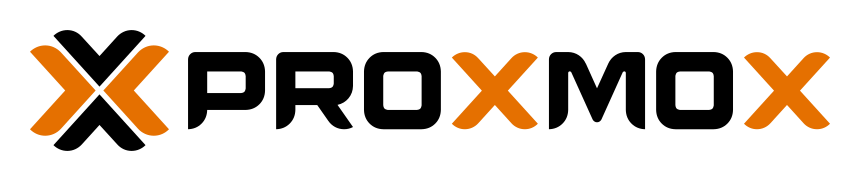
Proxmox Virtual Environment (Proxmox VE) v7.2.0 published on Sunday, Jun 29, 2025 by Daniel Muehlbachler-Pietrzykowski