Proxmox Virtual Environment (Proxmox VE) v7.0.0 published on Tuesday, Apr 1, 2025 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Metrics.getServer
Explore with Pulumi AI
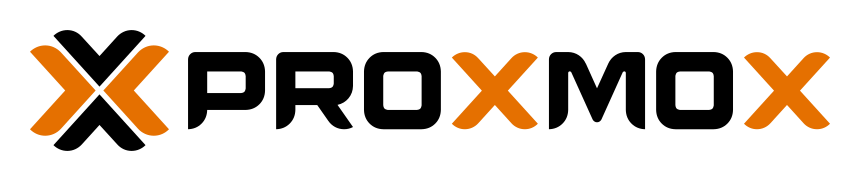
Proxmox Virtual Environment (Proxmox VE) v7.0.0 published on Tuesday, Apr 1, 2025 by Daniel Muehlbachler-Pietrzykowski
Retrieves information about a specific PVE metric server.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const example = proxmoxve.Metrics.getServer({
name: "example_influxdb",
});
export const dataProxmoxVirtualEnvironmentMetricsServer = {
server: example.then(example => example.server),
port: example.then(example => example.port),
};
import pulumi
import pulumi_proxmoxve as proxmoxve
example = proxmoxve.Metrics.get_server(name="example_influxdb")
pulumi.export("dataProxmoxVirtualEnvironmentMetricsServer", {
"server": example.server,
"port": example.port,
})
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/metrics"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
example, err := metrics.GetServer(ctx, &metrics.GetServerArgs{
Name: "example_influxdb",
}, nil)
if err != nil {
return err
}
ctx.Export("dataProxmoxVirtualEnvironmentMetricsServer", pulumi.Map{
"server": example.Server,
"port": example.Port,
})
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example = ProxmoxVE.Metrics.GetServer.Invoke(new()
{
Name = "example_influxdb",
});
return new Dictionary<string, object?>
{
["dataProxmoxVirtualEnvironmentMetricsServer"] =
{
{ "server", example.Apply(getServerResult => getServerResult.Server) },
{ "port", example.Apply(getServerResult => getServerResult.Port) },
},
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Metrics.MetricsFunctions;
import com.pulumi.proxmoxve.Metrics.inputs.GetServerArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var example = MetricsFunctions.getServer(GetServerArgs.builder()
.name("example_influxdb")
.build());
ctx.export("dataProxmoxVirtualEnvironmentMetricsServer", %!v(PANIC=Format method: runtime error: invalid memory address or nil pointer dereference));
}
}
variables:
example:
fn::invoke:
function: proxmoxve:Metrics:getServer
arguments:
name: example_influxdb
outputs:
dataProxmoxVirtualEnvironmentMetricsServer:
server: ${example.server}
port: ${example.port}
Using getServer
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getServer(args: GetServerArgs, opts?: InvokeOptions): Promise<GetServerResult>
function getServerOutput(args: GetServerOutputArgs, opts?: InvokeOptions): Output<GetServerResult>
def get_server(name: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetServerResult
def get_server_output(name: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetServerResult]
func GetServer(ctx *Context, args *GetServerArgs, opts ...InvokeOption) (*GetServerResult, error)
func GetServerOutput(ctx *Context, args *GetServerOutputArgs, opts ...InvokeOption) GetServerResultOutput
> Note: This function is named GetServer
in the Go SDK.
public static class GetServer
{
public static Task<GetServerResult> InvokeAsync(GetServerArgs args, InvokeOptions? opts = null)
public static Output<GetServerResult> Invoke(GetServerInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetServerResult> getServer(GetServerArgs args, InvokeOptions options)
public static Output<GetServerResult> getServer(GetServerArgs args, InvokeOptions options)
fn::invoke:
function: proxmoxve:Metrics/getServer:getServer
arguments:
# arguments dictionary
The following arguments are supported:
- Name string
- Unique name that will be ID of this metric server in PVE.
- Name string
- Unique name that will be ID of this metric server in PVE.
- name String
- Unique name that will be ID of this metric server in PVE.
- name string
- Unique name that will be ID of this metric server in PVE.
- name str
- Unique name that will be ID of this metric server in PVE.
- name String
- Unique name that will be ID of this metric server in PVE.
getServer Result
The following output properties are available:
- disable Boolean
- Indicates if the metric server is disabled.
- id String
- The unique identifier of this resource.
- name String
- Unique name that will be ID of this metric server in PVE.
- port Integer
- Server network port.
- server String
- Server dns name or IP address.
- type String
- Plugin type. Either
graphite
orinfluxdb
.
- disable boolean
- Indicates if the metric server is disabled.
- id string
- The unique identifier of this resource.
- name string
- Unique name that will be ID of this metric server in PVE.
- port number
- Server network port.
- server string
- Server dns name or IP address.
- type string
- Plugin type. Either
graphite
orinfluxdb
.
- disable Boolean
- Indicates if the metric server is disabled.
- id String
- The unique identifier of this resource.
- name String
- Unique name that will be ID of this metric server in PVE.
- port Number
- Server network port.
- server String
- Server dns name or IP address.
- type String
- Plugin type. Either
graphite
orinfluxdb
.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
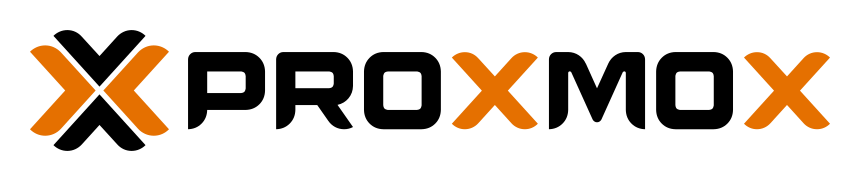
Proxmox Virtual Environment (Proxmox VE) v7.0.0 published on Tuesday, Apr 1, 2025 by Daniel Muehlbachler-Pietrzykowski