proxmoxve.Metrics.MetricsServer
Explore with Pulumi AI
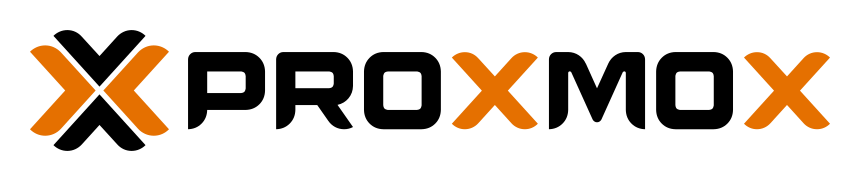
Manages PVE metrics server.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const influxdbServer = new proxmoxve.metrics.MetricsServer("influxdbServer", {
port: 8089,
server: "192.168.3.2",
type: "influxdb",
});
const graphiteServer = new proxmoxve.metrics.MetricsServer("graphiteServer", {
port: 2003,
server: "192.168.4.2",
type: "graphite",
});
import pulumi
import pulumi_proxmoxve as proxmoxve
influxdb_server = proxmoxve.metrics.MetricsServer("influxdbServer",
port=8089,
server="192.168.3.2",
type="influxdb")
graphite_server = proxmoxve.metrics.MetricsServer("graphiteServer",
port=2003,
server="192.168.4.2",
type="graphite")
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v7/go/proxmoxve/metrics"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := metrics.NewMetricsServer(ctx, "influxdbServer", &metrics.MetricsServerArgs{
Port: pulumi.Int(8089),
Server: pulumi.String("192.168.3.2"),
Type: pulumi.String("influxdb"),
})
if err != nil {
return err
}
_, err = metrics.NewMetricsServer(ctx, "graphiteServer", &metrics.MetricsServerArgs{
Port: pulumi.Int(2003),
Server: pulumi.String("192.168.4.2"),
Type: pulumi.String("graphite"),
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var influxdbServer = new ProxmoxVE.Metrics.MetricsServer("influxdbServer", new()
{
Port = 8089,
Server = "192.168.3.2",
Type = "influxdb",
});
var graphiteServer = new ProxmoxVE.Metrics.MetricsServer("graphiteServer", new()
{
Port = 2003,
Server = "192.168.4.2",
Type = "graphite",
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import io.muehlbachler.pulumi.proxmoxve.Metrics.MetricsServer;
import io.muehlbachler.pulumi.proxmoxve.Metrics.MetricsServerArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var influxdbServer = new MetricsServer("influxdbServer", MetricsServerArgs.builder()
.port(8089)
.server("192.168.3.2")
.type("influxdb")
.build());
var graphiteServer = new MetricsServer("graphiteServer", MetricsServerArgs.builder()
.port(2003)
.server("192.168.4.2")
.type("graphite")
.build());
}
}
resources:
influxdbServer:
type: proxmoxve:Metrics:MetricsServer
properties:
port: 8089
server: 192.168.3.2
type: influxdb
graphiteServer:
type: proxmoxve:Metrics:MetricsServer
properties:
port: 2003
server: 192.168.4.2
type: graphite
Create MetricsServer Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new MetricsServer(name: string, args: MetricsServerArgs, opts?: CustomResourceOptions);
@overload
def MetricsServer(resource_name: str,
args: MetricsServerArgs,
opts: Optional[ResourceOptions] = None)
@overload
def MetricsServer(resource_name: str,
opts: Optional[ResourceOptions] = None,
port: Optional[int] = None,
type: Optional[str] = None,
server: Optional[str] = None,
influx_bucket: Optional[str] = None,
disable: Optional[bool] = None,
influx_db_proto: Optional[str] = None,
influx_max_body_size: Optional[int] = None,
influx_organization: Optional[str] = None,
influx_token: Optional[str] = None,
influx_verify: Optional[bool] = None,
mtu: Optional[int] = None,
name: Optional[str] = None,
influx_api_path_prefix: Optional[str] = None,
graphite_proto: Optional[str] = None,
timeout: Optional[int] = None,
graphite_path: Optional[str] = None)
func NewMetricsServer(ctx *Context, name string, args MetricsServerArgs, opts ...ResourceOption) (*MetricsServer, error)
public MetricsServer(string name, MetricsServerArgs args, CustomResourceOptions? opts = null)
public MetricsServer(String name, MetricsServerArgs args)
public MetricsServer(String name, MetricsServerArgs args, CustomResourceOptions options)
type: proxmoxve:Metrics:MetricsServer
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args MetricsServerArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args MetricsServerArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args MetricsServerArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args MetricsServerArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args MetricsServerArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var metricsServerResource = new ProxmoxVE.Metrics.MetricsServer("metricsServerResource", new()
{
Port = 0,
Type = "string",
Server = "string",
InfluxBucket = "string",
Disable = false,
InfluxDbProto = "string",
InfluxMaxBodySize = 0,
InfluxOrganization = "string",
InfluxToken = "string",
InfluxVerify = false,
Mtu = 0,
Name = "string",
InfluxApiPathPrefix = "string",
GraphiteProto = "string",
Timeout = 0,
GraphitePath = "string",
});
example, err := metrics.NewMetricsServer(ctx, "metricsServerResource", &metrics.MetricsServerArgs{
Port: pulumi.Int(0),
Type: pulumi.String("string"),
Server: pulumi.String("string"),
InfluxBucket: pulumi.String("string"),
Disable: pulumi.Bool(false),
InfluxDbProto: pulumi.String("string"),
InfluxMaxBodySize: pulumi.Int(0),
InfluxOrganization: pulumi.String("string"),
InfluxToken: pulumi.String("string"),
InfluxVerify: pulumi.Bool(false),
Mtu: pulumi.Int(0),
Name: pulumi.String("string"),
InfluxApiPathPrefix: pulumi.String("string"),
GraphiteProto: pulumi.String("string"),
Timeout: pulumi.Int(0),
GraphitePath: pulumi.String("string"),
})
var metricsServerResource = new MetricsServer("metricsServerResource", MetricsServerArgs.builder()
.port(0)
.type("string")
.server("string")
.influxBucket("string")
.disable(false)
.influxDbProto("string")
.influxMaxBodySize(0)
.influxOrganization("string")
.influxToken("string")
.influxVerify(false)
.mtu(0)
.name("string")
.influxApiPathPrefix("string")
.graphiteProto("string")
.timeout(0)
.graphitePath("string")
.build());
metrics_server_resource = proxmoxve.metrics.MetricsServer("metricsServerResource",
port=0,
type="string",
server="string",
influx_bucket="string",
disable=False,
influx_db_proto="string",
influx_max_body_size=0,
influx_organization="string",
influx_token="string",
influx_verify=False,
mtu=0,
name="string",
influx_api_path_prefix="string",
graphite_proto="string",
timeout=0,
graphite_path="string")
const metricsServerResource = new proxmoxve.metrics.MetricsServer("metricsServerResource", {
port: 0,
type: "string",
server: "string",
influxBucket: "string",
disable: false,
influxDbProto: "string",
influxMaxBodySize: 0,
influxOrganization: "string",
influxToken: "string",
influxVerify: false,
mtu: 0,
name: "string",
influxApiPathPrefix: "string",
graphiteProto: "string",
timeout: 0,
graphitePath: "string",
});
type: proxmoxve:Metrics:MetricsServer
properties:
disable: false
graphitePath: string
graphiteProto: string
influxApiPathPrefix: string
influxBucket: string
influxDbProto: string
influxMaxBodySize: 0
influxOrganization: string
influxToken: string
influxVerify: false
mtu: 0
name: string
port: 0
server: string
timeout: 0
type: string
MetricsServer Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The MetricsServer resource accepts the following input properties:
- Port int
- Server network port.
- Server string
- Server dns name or IP address.
- Type string
- Plugin type. Choice is between
graphite
|influxdb
. - Disable bool
- Set this to
true
to disable this metric server. - Graphite
Path string - Root graphite path (ex:
proxmox.mycluster.mykey
). - Graphite
Proto string - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - Influx
Api stringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - Influx
Bucket string - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- Influx
Db stringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - Influx
Max intBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - Influx
Organization string - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- Influx
Token string - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - Influx
Verify bool - Set to
false
to disable certificate verification for https endpoints. - Mtu int
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - Name string
- Unique name that will be ID of this metric server in PVE.
- Timeout int
- TCP socket timeout in seconds. If not set, PVE default is
1
.
- Port int
- Server network port.
- Server string
- Server dns name or IP address.
- Type string
- Plugin type. Choice is between
graphite
|influxdb
. - Disable bool
- Set this to
true
to disable this metric server. - Graphite
Path string - Root graphite path (ex:
proxmox.mycluster.mykey
). - Graphite
Proto string - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - Influx
Api stringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - Influx
Bucket string - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- Influx
Db stringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - Influx
Max intBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - Influx
Organization string - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- Influx
Token string - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - Influx
Verify bool - Set to
false
to disable certificate verification for https endpoints. - Mtu int
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - Name string
- Unique name that will be ID of this metric server in PVE.
- Timeout int
- TCP socket timeout in seconds. If not set, PVE default is
1
.
- port Integer
- Server network port.
- server String
- Server dns name or IP address.
- type String
- Plugin type. Choice is between
graphite
|influxdb
. - disable Boolean
- Set this to
true
to disable this metric server. - graphite
Path String - Root graphite path (ex:
proxmox.mycluster.mykey
). - graphite
Proto String - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - influx
Api StringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - influx
Bucket String - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- influx
Db StringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - influx
Max IntegerBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - influx
Organization String - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- influx
Token String - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - influx
Verify Boolean - Set to
false
to disable certificate verification for https endpoints. - mtu Integer
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - name String
- Unique name that will be ID of this metric server in PVE.
- timeout Integer
- TCP socket timeout in seconds. If not set, PVE default is
1
.
- port number
- Server network port.
- server string
- Server dns name or IP address.
- type string
- Plugin type. Choice is between
graphite
|influxdb
. - disable boolean
- Set this to
true
to disable this metric server. - graphite
Path string - Root graphite path (ex:
proxmox.mycluster.mykey
). - graphite
Proto string - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - influx
Api stringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - influx
Bucket string - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- influx
Db stringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - influx
Max numberBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - influx
Organization string - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- influx
Token string - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - influx
Verify boolean - Set to
false
to disable certificate verification for https endpoints. - mtu number
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - name string
- Unique name that will be ID of this metric server in PVE.
- timeout number
- TCP socket timeout in seconds. If not set, PVE default is
1
.
- port int
- Server network port.
- server str
- Server dns name or IP address.
- type str
- Plugin type. Choice is between
graphite
|influxdb
. - disable bool
- Set this to
true
to disable this metric server. - graphite_
path str - Root graphite path (ex:
proxmox.mycluster.mykey
). - graphite_
proto str - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - influx_
api_ strpath_ prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - influx_
bucket str - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- influx_
db_ strproto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - influx_
max_ intbody_ size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - influx_
organization str - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- influx_
token str - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - influx_
verify bool - Set to
false
to disable certificate verification for https endpoints. - mtu int
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - name str
- Unique name that will be ID of this metric server in PVE.
- timeout int
- TCP socket timeout in seconds. If not set, PVE default is
1
.
- port Number
- Server network port.
- server String
- Server dns name or IP address.
- type String
- Plugin type. Choice is between
graphite
|influxdb
. - disable Boolean
- Set this to
true
to disable this metric server. - graphite
Path String - Root graphite path (ex:
proxmox.mycluster.mykey
). - graphite
Proto String - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - influx
Api StringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - influx
Bucket String - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- influx
Db StringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - influx
Max NumberBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - influx
Organization String - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- influx
Token String - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - influx
Verify Boolean - Set to
false
to disable certificate verification for https endpoints. - mtu Number
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - name String
- Unique name that will be ID of this metric server in PVE.
- timeout Number
- TCP socket timeout in seconds. If not set, PVE default is
1
.
Outputs
All input properties are implicitly available as output properties. Additionally, the MetricsServer resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing MetricsServer Resource
Get an existing MetricsServer resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: MetricsServerState, opts?: CustomResourceOptions): MetricsServer
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
disable: Optional[bool] = None,
graphite_path: Optional[str] = None,
graphite_proto: Optional[str] = None,
influx_api_path_prefix: Optional[str] = None,
influx_bucket: Optional[str] = None,
influx_db_proto: Optional[str] = None,
influx_max_body_size: Optional[int] = None,
influx_organization: Optional[str] = None,
influx_token: Optional[str] = None,
influx_verify: Optional[bool] = None,
mtu: Optional[int] = None,
name: Optional[str] = None,
port: Optional[int] = None,
server: Optional[str] = None,
timeout: Optional[int] = None,
type: Optional[str] = None) -> MetricsServer
func GetMetricsServer(ctx *Context, name string, id IDInput, state *MetricsServerState, opts ...ResourceOption) (*MetricsServer, error)
public static MetricsServer Get(string name, Input<string> id, MetricsServerState? state, CustomResourceOptions? opts = null)
public static MetricsServer get(String name, Output<String> id, MetricsServerState state, CustomResourceOptions options)
resources: _: type: proxmoxve:Metrics:MetricsServer get: id: ${id}
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Disable bool
- Set this to
true
to disable this metric server. - Graphite
Path string - Root graphite path (ex:
proxmox.mycluster.mykey
). - Graphite
Proto string - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - Influx
Api stringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - Influx
Bucket string - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- Influx
Db stringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - Influx
Max intBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - Influx
Organization string - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- Influx
Token string - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - Influx
Verify bool - Set to
false
to disable certificate verification for https endpoints. - Mtu int
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - Name string
- Unique name that will be ID of this metric server in PVE.
- Port int
- Server network port.
- Server string
- Server dns name or IP address.
- Timeout int
- TCP socket timeout in seconds. If not set, PVE default is
1
. - Type string
- Plugin type. Choice is between
graphite
|influxdb
.
- Disable bool
- Set this to
true
to disable this metric server. - Graphite
Path string - Root graphite path (ex:
proxmox.mycluster.mykey
). - Graphite
Proto string - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - Influx
Api stringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - Influx
Bucket string - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- Influx
Db stringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - Influx
Max intBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - Influx
Organization string - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- Influx
Token string - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - Influx
Verify bool - Set to
false
to disable certificate verification for https endpoints. - Mtu int
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - Name string
- Unique name that will be ID of this metric server in PVE.
- Port int
- Server network port.
- Server string
- Server dns name or IP address.
- Timeout int
- TCP socket timeout in seconds. If not set, PVE default is
1
. - Type string
- Plugin type. Choice is between
graphite
|influxdb
.
- disable Boolean
- Set this to
true
to disable this metric server. - graphite
Path String - Root graphite path (ex:
proxmox.mycluster.mykey
). - graphite
Proto String - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - influx
Api StringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - influx
Bucket String - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- influx
Db StringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - influx
Max IntegerBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - influx
Organization String - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- influx
Token String - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - influx
Verify Boolean - Set to
false
to disable certificate verification for https endpoints. - mtu Integer
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - name String
- Unique name that will be ID of this metric server in PVE.
- port Integer
- Server network port.
- server String
- Server dns name or IP address.
- timeout Integer
- TCP socket timeout in seconds. If not set, PVE default is
1
. - type String
- Plugin type. Choice is between
graphite
|influxdb
.
- disable boolean
- Set this to
true
to disable this metric server. - graphite
Path string - Root graphite path (ex:
proxmox.mycluster.mykey
). - graphite
Proto string - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - influx
Api stringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - influx
Bucket string - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- influx
Db stringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - influx
Max numberBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - influx
Organization string - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- influx
Token string - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - influx
Verify boolean - Set to
false
to disable certificate verification for https endpoints. - mtu number
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - name string
- Unique name that will be ID of this metric server in PVE.
- port number
- Server network port.
- server string
- Server dns name or IP address.
- timeout number
- TCP socket timeout in seconds. If not set, PVE default is
1
. - type string
- Plugin type. Choice is between
graphite
|influxdb
.
- disable bool
- Set this to
true
to disable this metric server. - graphite_
path str - Root graphite path (ex:
proxmox.mycluster.mykey
). - graphite_
proto str - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - influx_
api_ strpath_ prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - influx_
bucket str - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- influx_
db_ strproto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - influx_
max_ intbody_ size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - influx_
organization str - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- influx_
token str - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - influx_
verify bool - Set to
false
to disable certificate verification for https endpoints. - mtu int
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - name str
- Unique name that will be ID of this metric server in PVE.
- port int
- Server network port.
- server str
- Server dns name or IP address.
- timeout int
- TCP socket timeout in seconds. If not set, PVE default is
1
. - type str
- Plugin type. Choice is between
graphite
|influxdb
.
- disable Boolean
- Set this to
true
to disable this metric server. - graphite
Path String - Root graphite path (ex:
proxmox.mycluster.mykey
). - graphite
Proto String - Protocol to send graphite data. Choice is between
udp
|tcp
. If not set, PVE default isudp
. - influx
Api StringPath Prefix - An API path prefix inserted between
<host>:<port>/
and/api2/
. Can be useful if the InfluxDB service runs behind a reverse proxy. - influx
Bucket String - The InfluxDB bucket/db. Only necessary when using the http v2 api.
- influx
Db StringProto - Protocol for InfluxDB. Choice is between
udp
|http
|https
. If not set, PVE default isudp
. - influx
Max NumberBody Size - InfluxDB max-body-size in bytes. Requests are batched up to this size. If not set, PVE default is
25000000
. - influx
Organization String - The InfluxDB organization. Only necessary when using the http v2 api. Has no meaning when using v2 compatibility api.
- influx
Token String - The InfluxDB access token. Only necessary when using the http v2 api. If the v2 compatibility api is used, use
user:password
instead. - influx
Verify Boolean - Set to
false
to disable certificate verification for https endpoints. - mtu Number
- MTU (maximum transmission unit) for metrics transmission over UDP. If not set, PVE default is
1500
(allowed512
-65536
). - name String
- Unique name that will be ID of this metric server in PVE.
- port Number
- Server network port.
- server String
- Server dns name or IP address.
- timeout Number
- TCP socket timeout in seconds. If not set, PVE default is
1
. - type String
- Plugin type. Choice is between
graphite
|influxdb
.
Import
#!/usr/bin/env sh
$ pulumi import proxmoxve:Metrics/metricsServer:MetricsServer example example
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
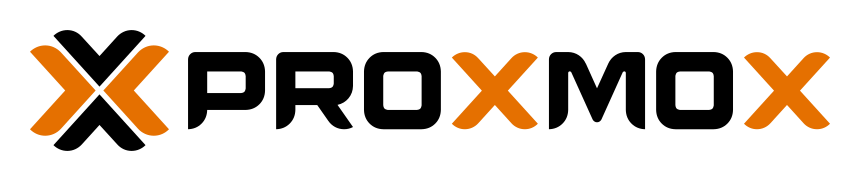