proxmoxve.Network.FirewallIPSet
Explore with Pulumi AI
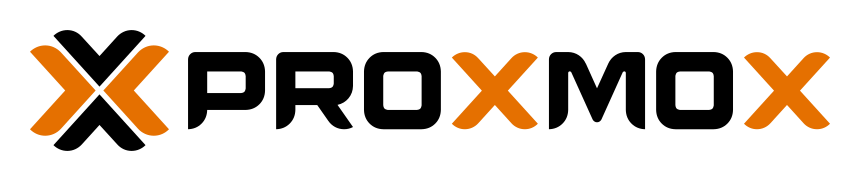
An IPSet allows us to group multiple IP addresses, IP subnets and aliases. Aliases can be created on the cluster level, on VM / Container level.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const ipset = new proxmoxve.network.FirewallIPSet("ipset", {
nodeName: proxmox_virtual_environment_vm.example.node_name,
vmId: proxmox_virtual_environment_vm.example.vm_id,
comment: "Managed by Terraform",
cidrs: [
{
name: "192.168.0.0/23",
comment: "Local network 1",
},
{
name: "192.168.0.1",
comment: "Server 1",
nomatch: true,
},
{
name: "192.168.2.1",
comment: "Server 1",
},
],
}, {
dependsOn: [proxmox_virtual_environment_vm.example],
});
import pulumi
import pulumi_proxmoxve as proxmoxve
ipset = proxmoxve.network.FirewallIPSet("ipset",
node_name=proxmox_virtual_environment_vm["example"]["node_name"],
vm_id=proxmox_virtual_environment_vm["example"]["vm_id"],
comment="Managed by Terraform",
cidrs=[
proxmoxve.network.FirewallIPSetCidrArgs(
name="192.168.0.0/23",
comment="Local network 1",
),
proxmoxve.network.FirewallIPSetCidrArgs(
name="192.168.0.1",
comment="Server 1",
nomatch=True,
),
proxmoxve.network.FirewallIPSetCidrArgs(
name="192.168.2.1",
comment="Server 1",
),
],
opts = pulumi.ResourceOptions(depends_on=[proxmox_virtual_environment_vm["example"]]))
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Network"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Network.NewFirewallIPSet(ctx, "ipset", &Network.FirewallIPSetArgs{
NodeName: pulumi.Any(proxmox_virtual_environment_vm.Example.Node_name),
VmId: pulumi.Any(proxmox_virtual_environment_vm.Example.Vm_id),
Comment: pulumi.String("Managed by Terraform"),
Cidrs: network.FirewallIPSetCidrArray{
&network.FirewallIPSetCidrArgs{
Name: pulumi.String("192.168.0.0/23"),
Comment: pulumi.String("Local network 1"),
},
&network.FirewallIPSetCidrArgs{
Name: pulumi.String("192.168.0.1"),
Comment: pulumi.String("Server 1"),
Nomatch: pulumi.Bool(true),
},
&network.FirewallIPSetCidrArgs{
Name: pulumi.String("192.168.2.1"),
Comment: pulumi.String("Server 1"),
},
},
}, pulumi.DependsOn([]pulumi.Resource{
proxmox_virtual_environment_vm.Example,
}))
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var ipset = new ProxmoxVE.Network.FirewallIPSet("ipset", new()
{
NodeName = proxmox_virtual_environment_vm.Example.Node_name,
VmId = proxmox_virtual_environment_vm.Example.Vm_id,
Comment = "Managed by Terraform",
Cidrs = new[]
{
new ProxmoxVE.Network.Inputs.FirewallIPSetCidrArgs
{
Name = "192.168.0.0/23",
Comment = "Local network 1",
},
new ProxmoxVE.Network.Inputs.FirewallIPSetCidrArgs
{
Name = "192.168.0.1",
Comment = "Server 1",
Nomatch = true,
},
new ProxmoxVE.Network.Inputs.FirewallIPSetCidrArgs
{
Name = "192.168.2.1",
Comment = "Server 1",
},
},
}, new CustomResourceOptions
{
DependsOn =
{
proxmox_virtual_environment_vm.Example,
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Network.FirewallIPSet;
import com.pulumi.proxmoxve.Network.FirewallIPSetArgs;
import com.pulumi.proxmoxve.Network.inputs.FirewallIPSetCidrArgs;
import com.pulumi.resources.CustomResourceOptions;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var ipset = new FirewallIPSet("ipset", FirewallIPSetArgs.builder()
.nodeName(proxmox_virtual_environment_vm.example().node_name())
.vmId(proxmox_virtual_environment_vm.example().vm_id())
.comment("Managed by Terraform")
.cidrs(
FirewallIPSetCidrArgs.builder()
.name("192.168.0.0/23")
.comment("Local network 1")
.build(),
FirewallIPSetCidrArgs.builder()
.name("192.168.0.1")
.comment("Server 1")
.nomatch(true)
.build(),
FirewallIPSetCidrArgs.builder()
.name("192.168.2.1")
.comment("Server 1")
.build())
.build(), CustomResourceOptions.builder()
.dependsOn(proxmox_virtual_environment_vm.example())
.build());
}
}
resources:
ipset:
type: proxmoxve:Network:FirewallIPSet
properties:
nodeName: ${proxmox_virtual_environment_vm.example.node_name}
vmId: ${proxmox_virtual_environment_vm.example.vm_id}
comment: Managed by Terraform
cidrs:
- name: 192.168.0.0/23
comment: Local network 1
- name: 192.168.0.1
comment: Server 1
nomatch: true
- name: 192.168.2.1
comment: Server 1
options:
dependson:
- ${proxmox_virtual_environment_vm.example}
Create FirewallIPSet Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new FirewallIPSet(name: string, args?: FirewallIPSetArgs, opts?: CustomResourceOptions);
@overload
def FirewallIPSet(resource_name: str,
args: Optional[FirewallIPSetArgs] = None,
opts: Optional[ResourceOptions] = None)
@overload
def FirewallIPSet(resource_name: str,
opts: Optional[ResourceOptions] = None,
cidrs: Optional[Sequence[_network.FirewallIPSetCidrArgs]] = None,
comment: Optional[str] = None,
container_id: Optional[int] = None,
name: Optional[str] = None,
node_name: Optional[str] = None,
vm_id: Optional[int] = None)
func NewFirewallIPSet(ctx *Context, name string, args *FirewallIPSetArgs, opts ...ResourceOption) (*FirewallIPSet, error)
public FirewallIPSet(string name, FirewallIPSetArgs? args = null, CustomResourceOptions? opts = null)
public FirewallIPSet(String name, FirewallIPSetArgs args)
public FirewallIPSet(String name, FirewallIPSetArgs args, CustomResourceOptions options)
type: proxmoxve:Network:FirewallIPSet
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args FirewallIPSetArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args FirewallIPSetArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args FirewallIPSetArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args FirewallIPSetArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args FirewallIPSetArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var firewallIPSetResource = new ProxmoxVE.Network.FirewallIPSet("firewallIPSetResource", new()
{
Cidrs = new[]
{
new ProxmoxVE.Network.Inputs.FirewallIPSetCidrArgs
{
Name = "string",
Comment = "string",
Nomatch = false,
},
},
Comment = "string",
ContainerId = 0,
Name = "string",
NodeName = "string",
VmId = 0,
});
example, err := Network.NewFirewallIPSet(ctx, "firewallIPSetResource", &Network.FirewallIPSetArgs{
Cidrs: network.FirewallIPSetCidrArray{
&network.FirewallIPSetCidrArgs{
Name: pulumi.String("string"),
Comment: pulumi.String("string"),
Nomatch: pulumi.Bool(false),
},
},
Comment: pulumi.String("string"),
ContainerId: pulumi.Int(0),
Name: pulumi.String("string"),
NodeName: pulumi.String("string"),
VmId: pulumi.Int(0),
})
var firewallIPSetResource = new FirewallIPSet("firewallIPSetResource", FirewallIPSetArgs.builder()
.cidrs(FirewallIPSetCidrArgs.builder()
.name("string")
.comment("string")
.nomatch(false)
.build())
.comment("string")
.containerId(0)
.name("string")
.nodeName("string")
.vmId(0)
.build());
firewall_ip_set_resource = proxmoxve.network.FirewallIPSet("firewallIPSetResource",
cidrs=[proxmoxve.network.FirewallIPSetCidrArgs(
name="string",
comment="string",
nomatch=False,
)],
comment="string",
container_id=0,
name="string",
node_name="string",
vm_id=0)
const firewallIPSetResource = new proxmoxve.network.FirewallIPSet("firewallIPSetResource", {
cidrs: [{
name: "string",
comment: "string",
nomatch: false,
}],
comment: "string",
containerId: 0,
name: "string",
nodeName: "string",
vmId: 0,
});
type: proxmoxve:Network:FirewallIPSet
properties:
cidrs:
- comment: string
name: string
nomatch: false
comment: string
containerId: 0
name: string
nodeName: string
vmId: 0
FirewallIPSet Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The FirewallIPSet resource accepts the following input properties:
- Cidrs
List<Pulumi.
Proxmox VE. Network. Inputs. Firewall IPSet Cidr> - IP/CIDR block (multiple blocks supported).
- Comment string
- IPSet comment.
- Container
Id int - Container ID. Leave empty for cluster level aliases.
- Name string
- IPSet name.
- Node
Name string - Node name. Leave empty for cluster level aliases.
- Vm
Id int - VM ID. Leave empty for cluster level aliases.
- Cidrs
[]Firewall
IPSet Cidr Args - IP/CIDR block (multiple blocks supported).
- Comment string
- IPSet comment.
- Container
Id int - Container ID. Leave empty for cluster level aliases.
- Name string
- IPSet name.
- Node
Name string - Node name. Leave empty for cluster level aliases.
- Vm
Id int - VM ID. Leave empty for cluster level aliases.
- cidrs
List<Firewall
IPSet Cidr> - IP/CIDR block (multiple blocks supported).
- comment String
- IPSet comment.
- container
Id Integer - Container ID. Leave empty for cluster level aliases.
- name String
- IPSet name.
- node
Name String - Node name. Leave empty for cluster level aliases.
- vm
Id Integer - VM ID. Leave empty for cluster level aliases.
- cidrs
Firewall
IPSet Cidr[] - IP/CIDR block (multiple blocks supported).
- comment string
- IPSet comment.
- container
Id number - Container ID. Leave empty for cluster level aliases.
- name string
- IPSet name.
- node
Name string - Node name. Leave empty for cluster level aliases.
- vm
Id number - VM ID. Leave empty for cluster level aliases.
- cidrs
Sequence[network.
Firewall IPSet Cidr Args] - IP/CIDR block (multiple blocks supported).
- comment str
- IPSet comment.
- container_
id int - Container ID. Leave empty for cluster level aliases.
- name str
- IPSet name.
- node_
name str - Node name. Leave empty for cluster level aliases.
- vm_
id int - VM ID. Leave empty for cluster level aliases.
- cidrs List<Property Map>
- IP/CIDR block (multiple blocks supported).
- comment String
- IPSet comment.
- container
Id Number - Container ID. Leave empty for cluster level aliases.
- name String
- IPSet name.
- node
Name String - Node name. Leave empty for cluster level aliases.
- vm
Id Number - VM ID. Leave empty for cluster level aliases.
Outputs
All input properties are implicitly available as output properties. Additionally, the FirewallIPSet resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing FirewallIPSet Resource
Get an existing FirewallIPSet resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: FirewallIPSetState, opts?: CustomResourceOptions): FirewallIPSet
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
cidrs: Optional[Sequence[_network.FirewallIPSetCidrArgs]] = None,
comment: Optional[str] = None,
container_id: Optional[int] = None,
name: Optional[str] = None,
node_name: Optional[str] = None,
vm_id: Optional[int] = None) -> FirewallIPSet
func GetFirewallIPSet(ctx *Context, name string, id IDInput, state *FirewallIPSetState, opts ...ResourceOption) (*FirewallIPSet, error)
public static FirewallIPSet Get(string name, Input<string> id, FirewallIPSetState? state, CustomResourceOptions? opts = null)
public static FirewallIPSet get(String name, Output<String> id, FirewallIPSetState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Cidrs
List<Pulumi.
Proxmox VE. Network. Inputs. Firewall IPSet Cidr> - IP/CIDR block (multiple blocks supported).
- Comment string
- IPSet comment.
- Container
Id int - Container ID. Leave empty for cluster level aliases.
- Name string
- IPSet name.
- Node
Name string - Node name. Leave empty for cluster level aliases.
- Vm
Id int - VM ID. Leave empty for cluster level aliases.
- Cidrs
[]Firewall
IPSet Cidr Args - IP/CIDR block (multiple blocks supported).
- Comment string
- IPSet comment.
- Container
Id int - Container ID. Leave empty for cluster level aliases.
- Name string
- IPSet name.
- Node
Name string - Node name. Leave empty for cluster level aliases.
- Vm
Id int - VM ID. Leave empty for cluster level aliases.
- cidrs
List<Firewall
IPSet Cidr> - IP/CIDR block (multiple blocks supported).
- comment String
- IPSet comment.
- container
Id Integer - Container ID. Leave empty for cluster level aliases.
- name String
- IPSet name.
- node
Name String - Node name. Leave empty for cluster level aliases.
- vm
Id Integer - VM ID. Leave empty for cluster level aliases.
- cidrs
Firewall
IPSet Cidr[] - IP/CIDR block (multiple blocks supported).
- comment string
- IPSet comment.
- container
Id number - Container ID. Leave empty for cluster level aliases.
- name string
- IPSet name.
- node
Name string - Node name. Leave empty for cluster level aliases.
- vm
Id number - VM ID. Leave empty for cluster level aliases.
- cidrs
Sequence[network.
Firewall IPSet Cidr Args] - IP/CIDR block (multiple blocks supported).
- comment str
- IPSet comment.
- container_
id int - Container ID. Leave empty for cluster level aliases.
- name str
- IPSet name.
- node_
name str - Node name. Leave empty for cluster level aliases.
- vm_
id int - VM ID. Leave empty for cluster level aliases.
- cidrs List<Property Map>
- IP/CIDR block (multiple blocks supported).
- comment String
- IPSet comment.
- container
Id Number - Container ID. Leave empty for cluster level aliases.
- name String
- IPSet name.
- node
Name String - Node name. Leave empty for cluster level aliases.
- vm
Id Number - VM ID. Leave empty for cluster level aliases.
Supporting Types
FirewallIPSetCidr, FirewallIPSetCidrArgs
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
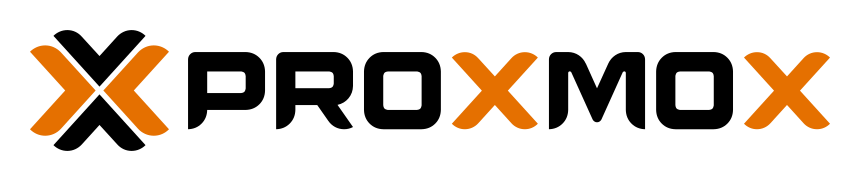