proxmoxve.Network.FirewallRules
Explore with Pulumi AI
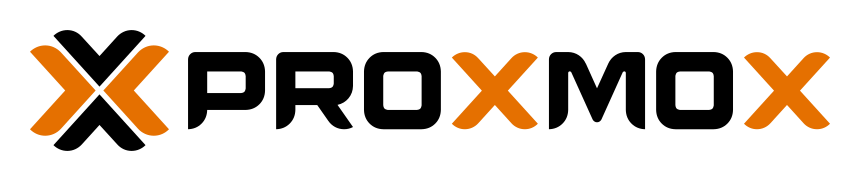
A security group is a collection of rules, defined at cluster level, which can be used in all VMs’ rules. For example, you can define a group named “webserver” with rules to open the http and https ports. Rules can be created on the cluster level, on VM / Container level.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const inbound = new proxmoxve.network.FirewallRules("inbound", {
nodeName: proxmox_virtual_environment_vm.example.node_name,
vmId: proxmox_virtual_environment_vm.example.vm_id,
rules: [
{
type: "in",
action: "ACCEPT",
comment: "Allow HTTP",
dest: "192.168.1.5",
dport: "80",
proto: "tcp",
log: "info",
},
{
type: "in",
action: "ACCEPT",
comment: "Allow HTTPS",
dest: "192.168.1.5",
dport: "443",
proto: "tcp",
log: "info",
},
{
securityGroup: proxmox_virtual_environment_cluster_firewall_security_group.example.name,
comment: "From security group",
iface: "net0",
},
],
}, {
dependsOn: [
proxmox_virtual_environment_vm.example,
proxmox_virtual_environment_cluster_firewall_security_group.example,
],
});
import pulumi
import pulumi_proxmoxve as proxmoxve
inbound = proxmoxve.network.FirewallRules("inbound",
node_name=proxmox_virtual_environment_vm["example"]["node_name"],
vm_id=proxmox_virtual_environment_vm["example"]["vm_id"],
rules=[
{
"type": "in",
"action": "ACCEPT",
"comment": "Allow HTTP",
"dest": "192.168.1.5",
"dport": "80",
"proto": "tcp",
"log": "info",
},
{
"type": "in",
"action": "ACCEPT",
"comment": "Allow HTTPS",
"dest": "192.168.1.5",
"dport": "443",
"proto": "tcp",
"log": "info",
},
{
"security_group": proxmox_virtual_environment_cluster_firewall_security_group["example"]["name"],
"comment": "From security group",
"iface": "net0",
},
],
opts = pulumi.ResourceOptions(depends_on=[
proxmox_virtual_environment_vm["example"],
proxmox_virtual_environment_cluster_firewall_security_group["example"],
]))
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v7/go/proxmoxve/network"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := network.NewFirewallRules(ctx, "inbound", &network.FirewallRulesArgs{
NodeName: pulumi.Any(proxmox_virtual_environment_vm.Example.Node_name),
VmId: pulumi.Any(proxmox_virtual_environment_vm.Example.Vm_id),
Rules: network.FirewallRulesRuleArray{
&network.FirewallRulesRuleArgs{
Type: pulumi.String("in"),
Action: pulumi.String("ACCEPT"),
Comment: pulumi.String("Allow HTTP"),
Dest: pulumi.String("192.168.1.5"),
Dport: pulumi.String("80"),
Proto: pulumi.String("tcp"),
Log: pulumi.String("info"),
},
&network.FirewallRulesRuleArgs{
Type: pulumi.String("in"),
Action: pulumi.String("ACCEPT"),
Comment: pulumi.String("Allow HTTPS"),
Dest: pulumi.String("192.168.1.5"),
Dport: pulumi.String("443"),
Proto: pulumi.String("tcp"),
Log: pulumi.String("info"),
},
&network.FirewallRulesRuleArgs{
SecurityGroup: pulumi.Any(proxmox_virtual_environment_cluster_firewall_security_group.Example.Name),
Comment: pulumi.String("From security group"),
Iface: pulumi.String("net0"),
},
},
}, pulumi.DependsOn([]pulumi.Resource{
proxmox_virtual_environment_vm.Example,
proxmox_virtual_environment_cluster_firewall_security_group.Example,
}))
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var inbound = new ProxmoxVE.Network.FirewallRules("inbound", new()
{
NodeName = proxmox_virtual_environment_vm.Example.Node_name,
VmId = proxmox_virtual_environment_vm.Example.Vm_id,
Rules = new[]
{
new ProxmoxVE.Network.Inputs.FirewallRulesRuleArgs
{
Type = "in",
Action = "ACCEPT",
Comment = "Allow HTTP",
Dest = "192.168.1.5",
Dport = "80",
Proto = "tcp",
Log = "info",
},
new ProxmoxVE.Network.Inputs.FirewallRulesRuleArgs
{
Type = "in",
Action = "ACCEPT",
Comment = "Allow HTTPS",
Dest = "192.168.1.5",
Dport = "443",
Proto = "tcp",
Log = "info",
},
new ProxmoxVE.Network.Inputs.FirewallRulesRuleArgs
{
SecurityGroup = proxmox_virtual_environment_cluster_firewall_security_group.Example.Name,
Comment = "From security group",
Iface = "net0",
},
},
}, new CustomResourceOptions
{
DependsOn =
{
proxmox_virtual_environment_vm.Example,
proxmox_virtual_environment_cluster_firewall_security_group.Example,
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import io.muehlbachler.pulumi.proxmoxve.Network.FirewallRules;
import io.muehlbachler.pulumi.proxmoxve.Network.FirewallRulesArgs;
import com.pulumi.proxmoxve.Network.inputs.FirewallRulesRuleArgs;
import com.pulumi.resources.CustomResourceOptions;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var inbound = new FirewallRules("inbound", FirewallRulesArgs.builder()
.nodeName(proxmox_virtual_environment_vm.example().node_name())
.vmId(proxmox_virtual_environment_vm.example().vm_id())
.rules(
FirewallRulesRuleArgs.builder()
.type("in")
.action("ACCEPT")
.comment("Allow HTTP")
.dest("192.168.1.5")
.dport("80")
.proto("tcp")
.log("info")
.build(),
FirewallRulesRuleArgs.builder()
.type("in")
.action("ACCEPT")
.comment("Allow HTTPS")
.dest("192.168.1.5")
.dport("443")
.proto("tcp")
.log("info")
.build(),
FirewallRulesRuleArgs.builder()
.securityGroup(proxmox_virtual_environment_cluster_firewall_security_group.example().name())
.comment("From security group")
.iface("net0")
.build())
.build(), CustomResourceOptions.builder()
.dependsOn(
proxmox_virtual_environment_vm.example(),
proxmox_virtual_environment_cluster_firewall_security_group.example())
.build());
}
}
resources:
inbound:
type: proxmoxve:Network:FirewallRules
properties:
nodeName: ${proxmox_virtual_environment_vm.example.node_name}
vmId: ${proxmox_virtual_environment_vm.example.vm_id}
rules:
- type: in
action: ACCEPT
comment: Allow HTTP
dest: 192.168.1.5
dport: '80'
proto: tcp
log: info
- type: in
action: ACCEPT
comment: Allow HTTPS
dest: 192.168.1.5
dport: '443'
proto: tcp
log: info
- securityGroup: ${proxmox_virtual_environment_cluster_firewall_security_group.example.name}
comment: From security group
iface: net0
options:
dependsOn:
- ${proxmox_virtual_environment_vm.example}
- ${proxmox_virtual_environment_cluster_firewall_security_group.example}
Create FirewallRules Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new FirewallRules(name: string, args: FirewallRulesArgs, opts?: CustomResourceOptions);
@overload
def FirewallRules(resource_name: str,
args: FirewallRulesArgs,
opts: Optional[ResourceOptions] = None)
@overload
def FirewallRules(resource_name: str,
opts: Optional[ResourceOptions] = None,
rules: Optional[Sequence[FirewallRulesRuleArgs]] = None,
container_id: Optional[int] = None,
node_name: Optional[str] = None,
vm_id: Optional[int] = None)
func NewFirewallRules(ctx *Context, name string, args FirewallRulesArgs, opts ...ResourceOption) (*FirewallRules, error)
public FirewallRules(string name, FirewallRulesArgs args, CustomResourceOptions? opts = null)
public FirewallRules(String name, FirewallRulesArgs args)
public FirewallRules(String name, FirewallRulesArgs args, CustomResourceOptions options)
type: proxmoxve:Network:FirewallRules
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args FirewallRulesArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args FirewallRulesArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args FirewallRulesArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args FirewallRulesArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args FirewallRulesArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var firewallRulesResource = new ProxmoxVE.Network.FirewallRules("firewallRulesResource", new()
{
Rules = new[]
{
new ProxmoxVE.Network.Inputs.FirewallRulesRuleArgs
{
Action = "string",
Comment = "string",
Dest = "string",
Dport = "string",
Enabled = false,
Iface = "string",
Log = "string",
Macro = "string",
Pos = 0,
Proto = "string",
SecurityGroup = "string",
Source = "string",
Sport = "string",
Type = "string",
},
},
ContainerId = 0,
NodeName = "string",
VmId = 0,
});
example, err := network.NewFirewallRules(ctx, "firewallRulesResource", &network.FirewallRulesArgs{
Rules: network.FirewallRulesRuleArray{
&network.FirewallRulesRuleArgs{
Action: pulumi.String("string"),
Comment: pulumi.String("string"),
Dest: pulumi.String("string"),
Dport: pulumi.String("string"),
Enabled: pulumi.Bool(false),
Iface: pulumi.String("string"),
Log: pulumi.String("string"),
Macro: pulumi.String("string"),
Pos: pulumi.Int(0),
Proto: pulumi.String("string"),
SecurityGroup: pulumi.String("string"),
Source: pulumi.String("string"),
Sport: pulumi.String("string"),
Type: pulumi.String("string"),
},
},
ContainerId: pulumi.Int(0),
NodeName: pulumi.String("string"),
VmId: pulumi.Int(0),
})
var firewallRulesResource = new FirewallRules("firewallRulesResource", FirewallRulesArgs.builder()
.rules(FirewallRulesRuleArgs.builder()
.action("string")
.comment("string")
.dest("string")
.dport("string")
.enabled(false)
.iface("string")
.log("string")
.macro("string")
.pos(0)
.proto("string")
.securityGroup("string")
.source("string")
.sport("string")
.type("string")
.build())
.containerId(0)
.nodeName("string")
.vmId(0)
.build());
firewall_rules_resource = proxmoxve.network.FirewallRules("firewallRulesResource",
rules=[{
"action": "string",
"comment": "string",
"dest": "string",
"dport": "string",
"enabled": False,
"iface": "string",
"log": "string",
"macro": "string",
"pos": 0,
"proto": "string",
"security_group": "string",
"source": "string",
"sport": "string",
"type": "string",
}],
container_id=0,
node_name="string",
vm_id=0)
const firewallRulesResource = new proxmoxve.network.FirewallRules("firewallRulesResource", {
rules: [{
action: "string",
comment: "string",
dest: "string",
dport: "string",
enabled: false,
iface: "string",
log: "string",
macro: "string",
pos: 0,
proto: "string",
securityGroup: "string",
source: "string",
sport: "string",
type: "string",
}],
containerId: 0,
nodeName: "string",
vmId: 0,
});
type: proxmoxve:Network:FirewallRules
properties:
containerId: 0
nodeName: string
rules:
- action: string
comment: string
dest: string
dport: string
enabled: false
iface: string
log: string
macro: string
pos: 0
proto: string
securityGroup: string
source: string
sport: string
type: string
vmId: 0
FirewallRules Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The FirewallRules resource accepts the following input properties:
- Rules
List<Pulumi.
Proxmox VE. Network. Inputs. Firewall Rules Rule> - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- Container
Id int - Container ID. Leave empty for cluster level rules.
- Node
Name string - Node name. Leave empty for cluster level rules.
- Vm
Id int - VM ID. Leave empty for cluster level rules.
- Rules
[]Firewall
Rules Rule Args - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- Container
Id int - Container ID. Leave empty for cluster level rules.
- Node
Name string - Node name. Leave empty for cluster level rules.
- Vm
Id int - VM ID. Leave empty for cluster level rules.
- rules
List<Firewall
Rules Rule> - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- container
Id Integer - Container ID. Leave empty for cluster level rules.
- node
Name String - Node name. Leave empty for cluster level rules.
- vm
Id Integer - VM ID. Leave empty for cluster level rules.
- rules
Firewall
Rules Rule[] - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- container
Id number - Container ID. Leave empty for cluster level rules.
- node
Name string - Node name. Leave empty for cluster level rules.
- vm
Id number - VM ID. Leave empty for cluster level rules.
- rules
Sequence[Firewall
Rules Rule Args] - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- container_
id int - Container ID. Leave empty for cluster level rules.
- node_
name str - Node name. Leave empty for cluster level rules.
- vm_
id int - VM ID. Leave empty for cluster level rules.
- rules List<Property Map>
- Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- container
Id Number - Container ID. Leave empty for cluster level rules.
- node
Name String - Node name. Leave empty for cluster level rules.
- vm
Id Number - VM ID. Leave empty for cluster level rules.
Outputs
All input properties are implicitly available as output properties. Additionally, the FirewallRules resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing FirewallRules Resource
Get an existing FirewallRules resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: FirewallRulesState, opts?: CustomResourceOptions): FirewallRules
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
container_id: Optional[int] = None,
node_name: Optional[str] = None,
rules: Optional[Sequence[FirewallRulesRuleArgs]] = None,
vm_id: Optional[int] = None) -> FirewallRules
func GetFirewallRules(ctx *Context, name string, id IDInput, state *FirewallRulesState, opts ...ResourceOption) (*FirewallRules, error)
public static FirewallRules Get(string name, Input<string> id, FirewallRulesState? state, CustomResourceOptions? opts = null)
public static FirewallRules get(String name, Output<String> id, FirewallRulesState state, CustomResourceOptions options)
resources: _: type: proxmoxve:Network:FirewallRules get: id: ${id}
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Container
Id int - Container ID. Leave empty for cluster level rules.
- Node
Name string - Node name. Leave empty for cluster level rules.
- Rules
List<Pulumi.
Proxmox VE. Network. Inputs. Firewall Rules Rule> - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- Vm
Id int - VM ID. Leave empty for cluster level rules.
- Container
Id int - Container ID. Leave empty for cluster level rules.
- Node
Name string - Node name. Leave empty for cluster level rules.
- Rules
[]Firewall
Rules Rule Args - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- Vm
Id int - VM ID. Leave empty for cluster level rules.
- container
Id Integer - Container ID. Leave empty for cluster level rules.
- node
Name String - Node name. Leave empty for cluster level rules.
- rules
List<Firewall
Rules Rule> - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- vm
Id Integer - VM ID. Leave empty for cluster level rules.
- container
Id number - Container ID. Leave empty for cluster level rules.
- node
Name string - Node name. Leave empty for cluster level rules.
- rules
Firewall
Rules Rule[] - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- vm
Id number - VM ID. Leave empty for cluster level rules.
- container_
id int - Container ID. Leave empty for cluster level rules.
- node_
name str - Node name. Leave empty for cluster level rules.
- rules
Sequence[Firewall
Rules Rule Args] - Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- vm_
id int - VM ID. Leave empty for cluster level rules.
- container
Id Number - Container ID. Leave empty for cluster level rules.
- node
Name String - Node name. Leave empty for cluster level rules.
- rules List<Property Map>
- Firewall rule block (multiple blocks supported).
The provider supports two types of the
rule
blocks:- A rule definition block, which includes the following arguments:
- vm
Id Number - VM ID. Leave empty for cluster level rules.
Supporting Types
FirewallRulesRule, FirewallRulesRuleArgs
- Action string
- Rule action (
ACCEPT
,DROP
,REJECT
). - Comment string
- Rule comment.
- Dest string
- Restrict packet destination address. This can
refer to a single IP address, an IP set ('+ipsetname') or an IP
alias definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - Dport string
- Restrict TCP/UDP destination port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges. - Enabled bool
- Enable this rule. Defaults to
true
. - Iface string
- Network interface name. You have to use network configuration key names for VMs and containers ('net\d+'). Host related rules can use arbitrary strings.
- Log string
- Log level for this rule (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Macro string
- Macro name. Use predefined standard macro from https://pve.proxmox.com/pve-docs/pve-admin-guide.html#_firewall_macro_definitions
- Pos int
- Position of the rule in the list.
- Proto string
- Restrict packet protocol. You can use protocol names as defined in '/etc/protocols'.
- Security
Group string - Security group name.
- Source string
- Restrict packet source address. This can refer
to a single IP address, an IP set ('+ipsetname') or an IP alias
definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - Sport string
- Restrict TCP/UDP source port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges.- a security group insertion block, which includes the following arguments:
- Type string
- Rule type (
in
,out
).
- Action string
- Rule action (
ACCEPT
,DROP
,REJECT
). - Comment string
- Rule comment.
- Dest string
- Restrict packet destination address. This can
refer to a single IP address, an IP set ('+ipsetname') or an IP
alias definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - Dport string
- Restrict TCP/UDP destination port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges. - Enabled bool
- Enable this rule. Defaults to
true
. - Iface string
- Network interface name. You have to use network configuration key names for VMs and containers ('net\d+'). Host related rules can use arbitrary strings.
- Log string
- Log level for this rule (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Macro string
- Macro name. Use predefined standard macro from https://pve.proxmox.com/pve-docs/pve-admin-guide.html#_firewall_macro_definitions
- Pos int
- Position of the rule in the list.
- Proto string
- Restrict packet protocol. You can use protocol names as defined in '/etc/protocols'.
- Security
Group string - Security group name.
- Source string
- Restrict packet source address. This can refer
to a single IP address, an IP set ('+ipsetname') or an IP alias
definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - Sport string
- Restrict TCP/UDP source port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges.- a security group insertion block, which includes the following arguments:
- Type string
- Rule type (
in
,out
).
- action String
- Rule action (
ACCEPT
,DROP
,REJECT
). - comment String
- Rule comment.
- dest String
- Restrict packet destination address. This can
refer to a single IP address, an IP set ('+ipsetname') or an IP
alias definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - dport String
- Restrict TCP/UDP destination port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges. - enabled Boolean
- Enable this rule. Defaults to
true
. - iface String
- Network interface name. You have to use network configuration key names for VMs and containers ('net\d+'). Host related rules can use arbitrary strings.
- log String
- Log level for this rule (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macro String
- Macro name. Use predefined standard macro from https://pve.proxmox.com/pve-docs/pve-admin-guide.html#_firewall_macro_definitions
- pos Integer
- Position of the rule in the list.
- proto String
- Restrict packet protocol. You can use protocol names as defined in '/etc/protocols'.
- security
Group String - Security group name.
- source String
- Restrict packet source address. This can refer
to a single IP address, an IP set ('+ipsetname') or an IP alias
definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - sport String
- Restrict TCP/UDP source port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges.- a security group insertion block, which includes the following arguments:
- type String
- Rule type (
in
,out
).
- action string
- Rule action (
ACCEPT
,DROP
,REJECT
). - comment string
- Rule comment.
- dest string
- Restrict packet destination address. This can
refer to a single IP address, an IP set ('+ipsetname') or an IP
alias definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - dport string
- Restrict TCP/UDP destination port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges. - enabled boolean
- Enable this rule. Defaults to
true
. - iface string
- Network interface name. You have to use network configuration key names for VMs and containers ('net\d+'). Host related rules can use arbitrary strings.
- log string
- Log level for this rule (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macro string
- Macro name. Use predefined standard macro from https://pve.proxmox.com/pve-docs/pve-admin-guide.html#_firewall_macro_definitions
- pos number
- Position of the rule in the list.
- proto string
- Restrict packet protocol. You can use protocol names as defined in '/etc/protocols'.
- security
Group string - Security group name.
- source string
- Restrict packet source address. This can refer
to a single IP address, an IP set ('+ipsetname') or an IP alias
definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - sport string
- Restrict TCP/UDP source port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges.- a security group insertion block, which includes the following arguments:
- type string
- Rule type (
in
,out
).
- action str
- Rule action (
ACCEPT
,DROP
,REJECT
). - comment str
- Rule comment.
- dest str
- Restrict packet destination address. This can
refer to a single IP address, an IP set ('+ipsetname') or an IP
alias definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - dport str
- Restrict TCP/UDP destination port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges. - enabled bool
- Enable this rule. Defaults to
true
. - iface str
- Network interface name. You have to use network configuration key names for VMs and containers ('net\d+'). Host related rules can use arbitrary strings.
- log str
- Log level for this rule (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macro str
- Macro name. Use predefined standard macro from https://pve.proxmox.com/pve-docs/pve-admin-guide.html#_firewall_macro_definitions
- pos int
- Position of the rule in the list.
- proto str
- Restrict packet protocol. You can use protocol names as defined in '/etc/protocols'.
- security_
group str - Security group name.
- source str
- Restrict packet source address. This can refer
to a single IP address, an IP set ('+ipsetname') or an IP alias
definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - sport str
- Restrict TCP/UDP source port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges.- a security group insertion block, which includes the following arguments:
- type str
- Rule type (
in
,out
).
- action String
- Rule action (
ACCEPT
,DROP
,REJECT
). - comment String
- Rule comment.
- dest String
- Restrict packet destination address. This can
refer to a single IP address, an IP set ('+ipsetname') or an IP
alias definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - dport String
- Restrict TCP/UDP destination port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges. - enabled Boolean
- Enable this rule. Defaults to
true
. - iface String
- Network interface name. You have to use network configuration key names for VMs and containers ('net\d+'). Host related rules can use arbitrary strings.
- log String
- Log level for this rule (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macro String
- Macro name. Use predefined standard macro from https://pve.proxmox.com/pve-docs/pve-admin-guide.html#_firewall_macro_definitions
- pos Number
- Position of the rule in the list.
- proto String
- Restrict packet protocol. You can use protocol names as defined in '/etc/protocols'.
- security
Group String - Security group name.
- source String
- Restrict packet source address. This can refer
to a single IP address, an IP set ('+ipsetname') or an IP alias
definition. You can also specify an address range
like
20.34.101.207-201.3.9.99
, or a list of IP addresses and networks (entries are separated by comma). Please do not mix IPv4 and IPv6 addresses inside such lists. - sport String
- Restrict TCP/UDP source port. You can use
service names or simple numbers (0-65535), as defined
in
/etc/services
. Port ranges can be specified with '\d+:\d+', for example80:85
, and you can use comma separated list to match several ports or ranges.- a security group insertion block, which includes the following arguments:
- type String
- Rule type (
in
,out
).
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
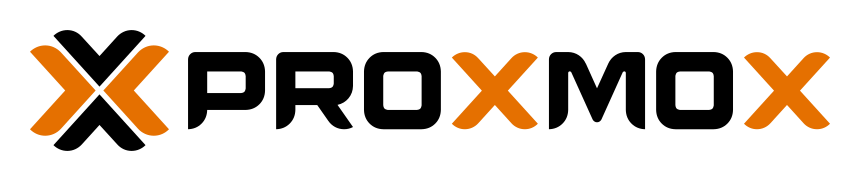