Proxmox Virtual Environment (Proxmox VE) v7.2.0 published on Sunday, Jun 29, 2025 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Permission.getGroup
Explore with Pulumi AI
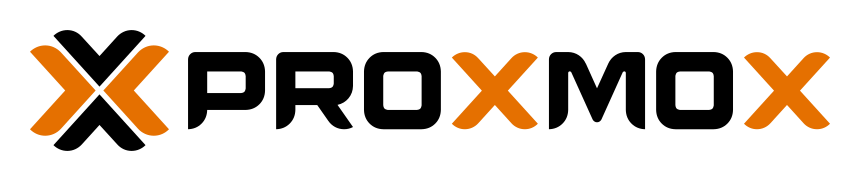
Proxmox Virtual Environment (Proxmox VE) v7.2.0 published on Sunday, Jun 29, 2025 by Daniel Muehlbachler-Pietrzykowski
Retrieves information about a specific user group.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const operationsTeam = proxmoxve.Permission.getGroup({
groupId: "operations-team",
});
import pulumi
import pulumi_proxmoxve as proxmoxve
operations_team = proxmoxve.Permission.get_group(group_id="operations-team")
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v7/go/proxmoxve/permission"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := permission.GetGroup(ctx, &permission.GetGroupArgs{
GroupId: "operations-team",
}, nil)
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var operationsTeam = ProxmoxVE.Permission.GetGroup.Invoke(new()
{
GroupId = "operations-team",
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Permission.PermissionFunctions;
import com.pulumi.proxmoxve.Permission.inputs.GetGroupArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var operationsTeam = PermissionFunctions.getGroup(GetGroupArgs.builder()
.groupId("operations-team")
.build());
}
}
variables:
operationsTeam:
fn::invoke:
function: proxmoxve:Permission:getGroup
arguments:
groupId: operations-team
Using getGroup
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getGroup(args: GetGroupArgs, opts?: InvokeOptions): Promise<GetGroupResult>
function getGroupOutput(args: GetGroupOutputArgs, opts?: InvokeOptions): Output<GetGroupResult>
def get_group(group_id: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetGroupResult
def get_group_output(group_id: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetGroupResult]
func LookupGroup(ctx *Context, args *LookupGroupArgs, opts ...InvokeOption) (*LookupGroupResult, error)
func LookupGroupOutput(ctx *Context, args *LookupGroupOutputArgs, opts ...InvokeOption) LookupGroupResultOutput
> Note: This function is named LookupGroup
in the Go SDK.
public static class GetGroup
{
public static Task<GetGroupResult> InvokeAsync(GetGroupArgs args, InvokeOptions? opts = null)
public static Output<GetGroupResult> Invoke(GetGroupInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetGroupResult> getGroup(GetGroupArgs args, InvokeOptions options)
public static Output<GetGroupResult> getGroup(GetGroupArgs args, InvokeOptions options)
fn::invoke:
function: proxmoxve:Permission/getGroup:getGroup
arguments:
# arguments dictionary
The following arguments are supported:
- Group
Id string - The group identifier.
- Group
Id string - The group identifier.
- group
Id String - The group identifier.
- group
Id string - The group identifier.
- group_
id str - The group identifier.
- group
Id String - The group identifier.
getGroup Result
The following output properties are available:
Supporting Types
GetGroupAcl
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
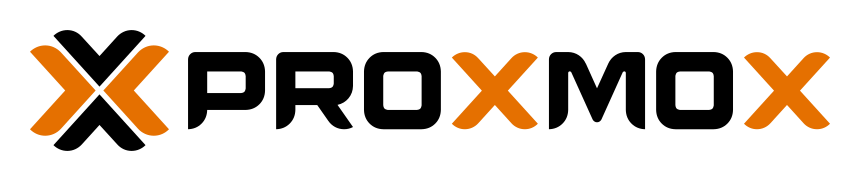
Proxmox Virtual Environment (Proxmox VE) v7.2.0 published on Sunday, Jun 29, 2025 by Daniel Muehlbachler-Pietrzykowski