Proxmox Virtual Environment (Proxmox VE) v6.4.1 published on Sunday, Apr 21, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Permission.getPool
Explore with Pulumi AI
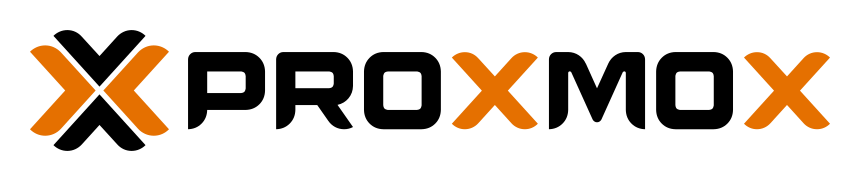
Proxmox Virtual Environment (Proxmox VE) v6.4.1 published on Sunday, Apr 21, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves information about a specific resource pool.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const operationsPool = proxmoxve.Permission.getPool({
poolId: "operations",
});
import pulumi
import pulumi_proxmoxve as proxmoxve
operations_pool = proxmoxve.Permission.get_pool(pool_id="operations")
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Permission"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Permission.GetPool(ctx, &permission.GetPoolArgs{
PoolId: "operations",
}, nil)
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var operationsPool = ProxmoxVE.Permission.GetPool.Invoke(new()
{
PoolId = "operations",
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Permission.PermissionFunctions;
import com.pulumi.proxmoxve.Permission.inputs.GetPoolArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var operationsPool = PermissionFunctions.getPool(GetPoolArgs.builder()
.poolId("operations")
.build());
}
}
variables:
operationsPool:
fn::invoke:
Function: proxmoxve:Permission:getPool
Arguments:
poolId: operations
Using getPool
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getPool(args: GetPoolArgs, opts?: InvokeOptions): Promise<GetPoolResult>
function getPoolOutput(args: GetPoolOutputArgs, opts?: InvokeOptions): Output<GetPoolResult>
def get_pool(pool_id: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetPoolResult
def get_pool_output(pool_id: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetPoolResult]
func GetPool(ctx *Context, args *GetPoolArgs, opts ...InvokeOption) (*GetPoolResult, error)
func GetPoolOutput(ctx *Context, args *GetPoolOutputArgs, opts ...InvokeOption) GetPoolResultOutput
> Note: This function is named GetPool
in the Go SDK.
public static class GetPool
{
public static Task<GetPoolResult> InvokeAsync(GetPoolArgs args, InvokeOptions? opts = null)
public static Output<GetPoolResult> Invoke(GetPoolInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetPoolResult> getPool(GetPoolArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:Permission/getPool:getPool
arguments:
# arguments dictionary
The following arguments are supported:
- Pool
Id string - The pool identifier.
- Pool
Id string - The pool identifier.
- pool
Id String - The pool identifier.
- pool
Id string - The pool identifier.
- pool_
id str - The pool identifier.
- pool
Id String - The pool identifier.
getPool Result
The following output properties are available:
- Comment string
- The pool comment.
- Id string
- The provider-assigned unique ID for this managed resource.
- Members
List<Pulumi.
Proxmox VE. Permission. Outputs. Get Pool Member> - The pool members.
- Pool
Id string
- Comment string
- The pool comment.
- Id string
- The provider-assigned unique ID for this managed resource.
- Members
[]Get
Pool Member - The pool members.
- Pool
Id string
- comment String
- The pool comment.
- id String
- The provider-assigned unique ID for this managed resource.
- members
List<Get
Pool Member> - The pool members.
- pool
Id String
- comment string
- The pool comment.
- id string
- The provider-assigned unique ID for this managed resource.
- members
Get
Pool Member[] - The pool members.
- pool
Id string
- comment str
- The pool comment.
- id str
- The provider-assigned unique ID for this managed resource.
- members
Sequence[permission.
Get Pool Member] - The pool members.
- pool_
id str
- comment String
- The pool comment.
- id String
- The provider-assigned unique ID for this managed resource.
- members List<Property Map>
- The pool members.
- pool
Id String
Supporting Types
GetPoolMember
- Datastore
Id string - The datastore identifier.
- Id string
- The member identifier.
- Node
Name string - The node name.
- Type string
- The member type.
- Vm
Id int - The virtual machine identifier.
- Datastore
Id string - The datastore identifier.
- Id string
- The member identifier.
- Node
Name string - The node name.
- Type string
- The member type.
- Vm
Id int - The virtual machine identifier.
- datastore
Id String - The datastore identifier.
- id String
- The member identifier.
- node
Name String - The node name.
- type String
- The member type.
- vm
Id Integer - The virtual machine identifier.
- datastore
Id string - The datastore identifier.
- id string
- The member identifier.
- node
Name string - The node name.
- type string
- The member type.
- vm
Id number - The virtual machine identifier.
- datastore_
id str - The datastore identifier.
- id str
- The member identifier.
- node_
name str - The node name.
- type str
- The member type.
- vm_
id int - The virtual machine identifier.
- datastore
Id String - The datastore identifier.
- id String
- The member identifier.
- node
Name String - The node name.
- type String
- The member type.
- vm
Id Number - The virtual machine identifier.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
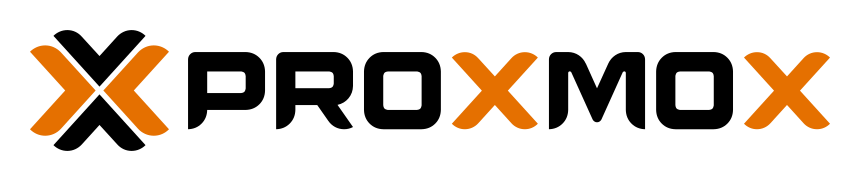
Proxmox Virtual Environment (Proxmox VE) v6.4.1 published on Sunday, Apr 21, 2024 by Daniel Muehlbachler-Pietrzykowski