Increase productivity with programming languages
AWS CloudFormation provides a configuration DSL for you to describe and provision infrastructure resources on AWS.
Pulumi enables you to describe the same infrastructure resources as real code, providing huge productivity gains, while decreasing the brittleness of YAML-based configuration files.
The benefits of using Pulumi
Tame cloud complexity
Deliver infrastructure from 50+ cloud and SaaS providers. Pulumi’s SDKs provide a complete and consistent interface that offers full access to clouds and abstracts complexity.
Bring the cloud closer to application development
Build reusable cloud infrastructure and infrastructure platforms that empower developers to build modern cloud applications faster and with less overhead.
Use engineering practices with infrastructure
Use engineering practices with infrastructure” to: “Replace inefficient, manual infrastructure processes with automation. Test and deliver infrastructure through CI/CD workflows or automate deployments with code at runtime.
Foster collaboration and innovate faster
Unite infrastructure teams, developers, and security teams around shared languages and tools so that everyone can ship products quickly and reliably.
Creating a Simple Web Server on AWS
In this example, we use JavaScript to create a simple web server on AWS using EC2.
This code creates the necessary security group, deploys a very simple web server for example purposes, and then creates the instance, before exporting the IP and hostname.
Pulumi can be used on any resource on AWS, Azure, Google Cloud, Kubernetes, and OpenStack, covering serverless, containers, and infrastructure.
const aws = require("@pulumi/aws");
let size = "t2.micro";
let ami = "ami-7172b611"
// Create a new security group for port 80.
let group = new aws.ec2.SecurityGroup("web-secgrp", {
ingress: [
{ protocol: "tcp", fromPort: 22,
toPort: 22, cidrBlocks: ["0.0.0.0/0"] },
{ protocol: "tcp", fromPort: 80,
toPort: 80, cidrBlocks: ["0.0.0.0/0"] },
],
});
// Create a simple web server.
let userData =
"#!/bin/bash \n" +
"echo 'Hello, World!' > index.html \n" +
"nohup python -m SimpleHTTPServer 80 &";
let server = new aws.ec2.Instance("web-server-www", {
tags: { "Name": "web-server-www" },
instanceType: size,
securityGroups: [ group.name ],
ami: ami,
userData: userData
});
exports.publicIp = server.publicIp;
exports.publicHostName = server.publicDns;
How Pulumi Works
Build
- Code in modern languages
- Share and reuse patterns
- Use your favorite IDE and tools
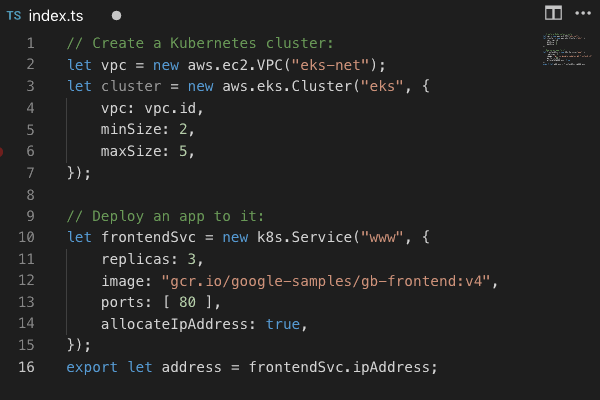
Deploy
- Preview changes
- Run
pulumi up
to deploy - Integrate with CI/CD
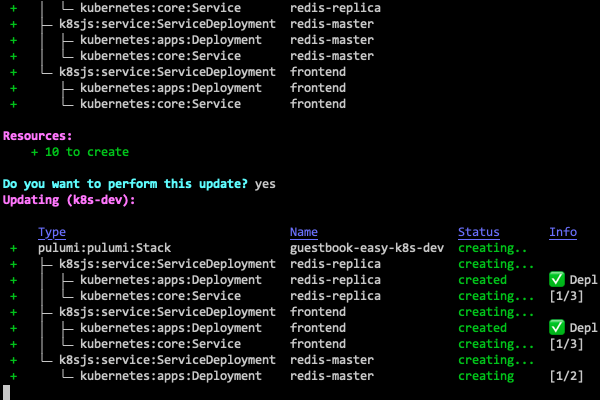
Manage
- Audit all changes
- Manage complex environments
- Implement policies and controls
Featured Customer
Hyland
Hyland, a blockchain SaaS company faced two challenges with their cloud infrastructure:
- Skills gaps between Dev and DevOps creating silos, and fragility.
- The need to more rapidly provision their expanding roster of new customers.
By moving to Pulumi, Hyland were able to solve both challenges with significant increases in capability:
Pulumi has given our team the tools and framework to achieve a unified development and DevOps model, boosting productivity and taking our business to any cloud environment that our customers need. We retired 25,000 lines of complex code that few team members understood and replaced it with 100s of lines in a real programming language.
Kim Hamilton
CTO
Get Started with Pulumi
Use Pulumi's open source SDK to create, deploy, and manage infrastructure on any cloud.