Universal Infrastructure as Code for AWS
Pulumi’s infrastructure as code SDK enables you to build, deploy, and manage your AWS infrastructure faster and with more confidence, using modern programming languages and software engineering practices. Leverage the full expressivity of general-purpose languages (TypeScript/JavaScript, Python, Go, C#, Java or use markup languages (e.g., YAML, CUE) to build any cloud architecture including containers, serverless, and server-based.
Build
Use familiar software practices and tools to build cloud infrastructure.
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of familiar programming languages and tools.
IDE Autocomplete
With Pulumi, you can use your preferred IDE and productivity features like autocompletion so that you author infrastructure code faster and with more accuracy.
Native Testing Frameworks
Use your favorite programming languages and popular testing frameworks to validate your infrastructure and applications through their entire lifecycle. Pulumi can also mock cloud resources for you so that you can perform offline testing that’s faster and cheaper.
Sharing and Reuse
Sharing and reusing your infrastructure code is available right out of the box with Pulumi Packages. You can use your preferred programming language's native package managers to share and distribute infrastructure code within your organization. Or, browse publicly available packages in Pulumi Registry.
Deploy
Increase automation and collaboration to ship faster.
Getting an application into production can be an overwhelming process. Pulumi empowers you and your team to work together to reduce obstacles and increase collaboration across your entire software delivery pipeline.
Type Name Status
+ pulumi:pulumi:Stack aws-typescript-dev created
+ └─ aws:s3:Bucket my-bucket created
bucketName: "my-bucket-9dfc488"
+ 2 created
8s
Full Coverage of AWS
You can deploy the entire breadth of AWS services with Pulumi’s AWS Classic Provider and Native Provider SDKs. The newly released AWS Native Provider (Preview) uses the AWS Cloud Control API to provide direct integration with AWS resource models and same-day updates for newly released AWS features.
Architect for self-service
Pulumi's unique approach to Infrastructure as Code allows you to build self-service infrastructure platforms. You can abstract away complexity for your teammates, allowing folks to focus on what's important.
Continuous delivery for infrastructure
Pulumi gives you the power to continuously test and deliver your cloud infrastructure by integrating with your favorite CI/CD platforms. By automating your testing and delivery you can focus more on delivering value to your customers.
Manage
Gain full auditability and visibility into all your environments to tame complexity and put security first.
Manage cloud infrastructure with dashboards that provide visibility into your infrastructure and any changes, role-based access controls, and Policy as Code enforcement across your organization.
Secrets management made easy
Pulumi provides you with any easy way to ensure secrets are kept safe during the entire software development lifecycle. You can have Pulumi manage your secrets for you or bring your own secrets management provider.
Keep your workflows secure
Enable Policy as Code within your organization so that you can define guardrails for your infrastructure, ensuring engineers are following best practices and putting security first. This helps you prevent mistakes before they occur and respond rapidly to any incidents.
Clear visibility across AWS resources
The Pulumi Service gives you full visibility into your cloud infrastructure’s current and past states. See every resource running in each stack with deep links to the AWS Console, actions performed by team members, Git-like diffs for infrastructure changes, and much more.
Trusted by top engineering teams
Programmatic Infrastructure as Code
Pulumi Automation API exposes the full power of infrastructure as code through a programmatic interface, instead of through CLI commands. Automation API lets you use the Pulumi engine as an SDK, enabling you to create software that can create, update, configure, and destroy infrastructure dynamically. This enables you to build custom cloud interfaces that are tailored to your team, organization, or customers.
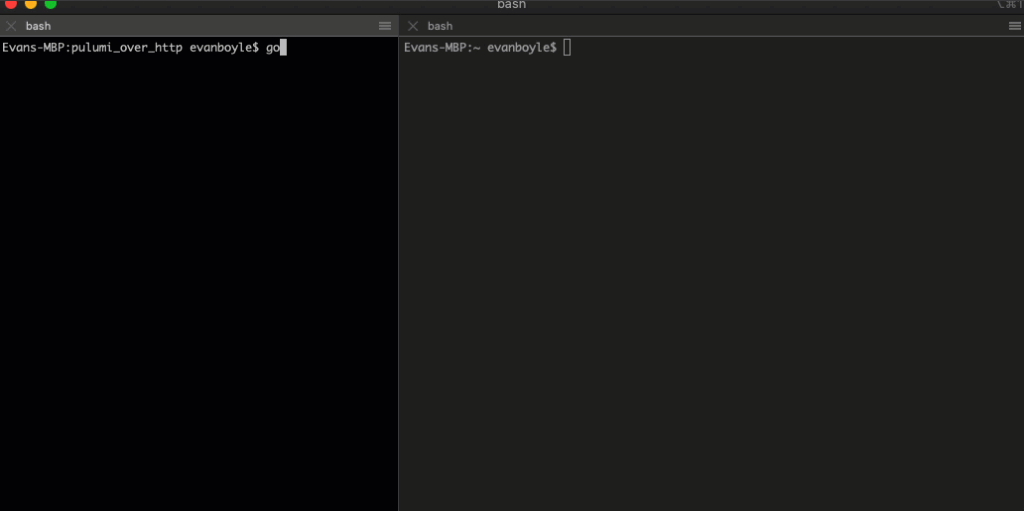
import * as eks from "@pulumi/eks";
// Create an EKS cluster.
const cluster = new eks.Cluster("cluster", {
instanceType: "t2.medium",
desiredCapacity: 2,
minSize: 1,
maxSize: 2,
});
// Export the cluster's kubeconfig.
export const kubeconfig = cluster.kubeconfig;
import pulumi
import pulumi_eks as eks
# Create an EKS cluster.
cluster = eks.Cluster(
"cluster",
instance_type="t2.medium",
desired_capacity=2,
min_size=1,
max_size=2,
)
# Export the cluster's kubeconfig.
pulumi.export("kubeconfig", cluster.kubeconfig)
package main
import (
"github.com/pulumi/pulumi-eks/sdk/go/eks/cluster"
"github.com/pulumi/pulumi/sdk/v2/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
// Create an EKS cluster.
cluster, err := cluster.NewCluster(ctx, "cluster",
cluster.ClusterArgs{
InstanceType: pulumi.String("t2.medium"),
DesiredCapacity: pulumi.Int(2),
MinSize: pulumi.Int(1),
MaxSize: pulumi.Int(2),
},
)
if err != nil {
return err
}
// Export the cluster's kubeconfig.
ctx.Export("kubeconfig", cluster.Kubeconfig)
return nil
})
}
using System;
using System.Threading.Tasks;
using Pulumi;
using Pulumi.Eks.Cluster;
class EksStack : Stack
{
public EksStack()
{
// Create an EKS cluster.
var cluster = new Cluster("cluster", new ClusterArgs
{
InstanceType = "t2.medium",
DesiredCapacity = 2,
MinSize = 1,
MaxSize = 2,
});
// Export the cluster's kubeconfig.
this.Kubeconfig = cluster.Kubeconfig;
}
[Output("kubeconfig")]
public Output<string> Kubeconfig { get; set; }
}
class Program
{
static Task<int> Main(string[] args) => Deployment.RunAsync<EksStack>();
}
package com.pulumi.example.eks;
import com.pulumi.Context;
import com.pulumi.Exports;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.eks.Cluster;
import com.pulumi.eks.ClusterArgs;
import java.util.stream.Collectors;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
private static Exports stack(Context ctx) {
var cluster = new Cluster("my-cluster", ClusterArgs.builder()
.instanceType("t2.micro")
.desiredCapacity(2)
.minSize(1)
.maxSize(2)
.build());
ctx.export("kubeconfig", cluster.kubeconfig());
return ctx.exports();
}
}
name: aws-eks
runtime: yaml
description: An EKS cluster
resources:
cluster:
type: eks:Cluster
properties:
instanceType: "t2.medium"
desiredCapacity: 2
minSize: 1
maxSize: 2
outputs:
kubeconfig: ${cluster.kubeconfig}
Use Well-Architected Practices
Pulumi Crosswalk for AWS is a collection of libraries that use automatic well-architected best practices to make common infrastructure-as-code tasks in AWS easier and more secure. Secure and cost-conscious defaults are chosen so that simple programs automatically use best practices for the underlying infrastructure, enabling better productivity with confidence.
Deploy CDK Constructs with Pulumi
You can deploy any AWS CDK construct from within a Pulumi deployment. If you're already using AWS CDK, you can now use Pulumi to orchestrate deployments instead of CloudFormation. This gives you [improved deployment speed](/case-studies/panther-labs/#proving-pulumis-advantages/) and integration with all features of Pulumi (like [Policy as Code](/docs/using-pulumi/crossguard/), [Audit Logs](/docs/pulumi-cloud/audit-logs/), Secrets, and much more).
Application and Infrastructure Together
Crosswalk enables you to blur the lines between application and infrastructure code enabling you to author an entire full-stack application in one program. With support for inline Lambda functions and ease-of-use helper functions, building robust applications on AWS has never been easier.
Watch a workshop
Get Started
Get started now
Deploy your first app in just five minutes. Follow our tutorials for AWS, Azure, Google Cloud, Kubernetes, and more.
Get StartedMigrating from other tools
Transition to Pulumi with converter tools for Terraform, AWS CloudFormation, Azure Resource Manager, and Kubernetes.
Explore Convertor Tools