Kubernetes Superpowers
Pulumi empowers organizations to automate Kubernetes applications, clusters, and entire cloud environments through code, tame secrets sprawl through centralized secrets management, and manage cloud assets and compliance with the help of AI. Pulumi encourages infrastructure, platform, development, DevOps, and security teams to collaborate and accelerates time to market with greater control and minimized risk.
Powering top engineering teams
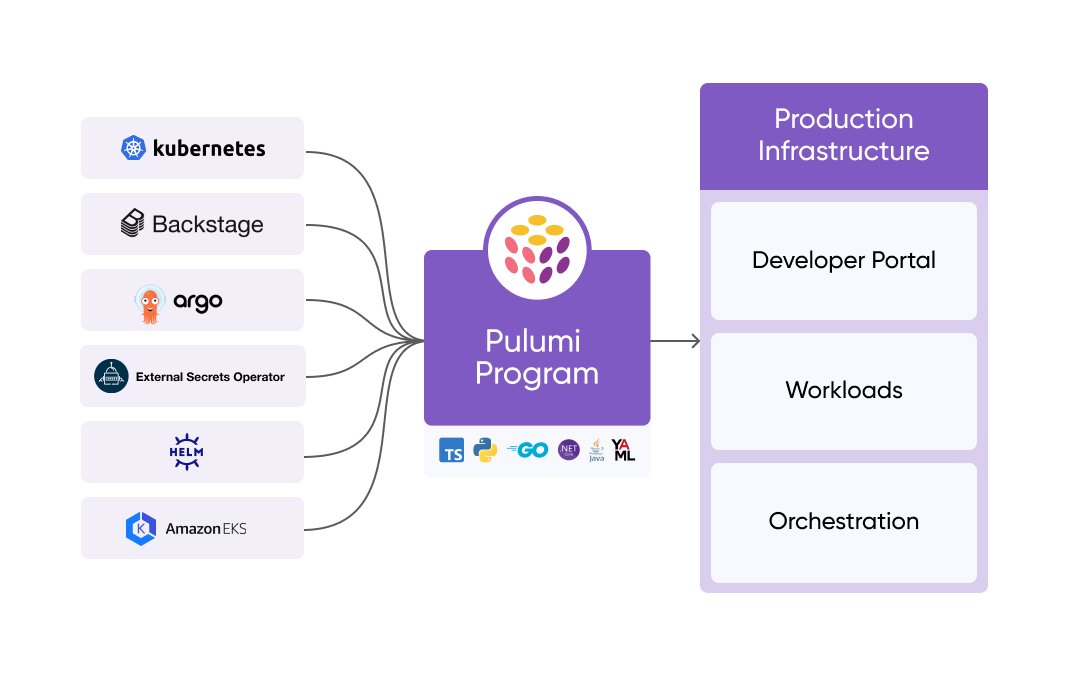
Cloud Native Engineering with Pulumi
Pulumi Infrastructure as Code (IaC) streamlines Kubernetes cluster configuration, management, and app workload deployments to your clusters. With Pulumi for Kubernetes you can:
- Manage Kubernetes clusters on all major cloud providers.
- Increase productivity using the full ecosystem of dev tools such as IDE auto-completion, type & error checking, linting, refactoring, and test frameworks to validate Kubernetes clusters, app workloads, or both.
- Automate Kubernetes deployments with CI/CD integrations for Flux, Spinnaker, Octopus, GitHub Actions, GitLab, Azure DevOps and more.
- Seamlessly manage both Kubernetes and cloud resources using GitOps with the Pulumi Kubernetes Operator, including ArgoCD integration.
- Use Kubernetes Server-Side Apply to safely manage shared Kubernetes resources with Pulumi and your existing controllers.
Infrastructure as Code for Kubernetes
Define your Kubernetes resources using the programming languages you know and love. With Pulumi's SDK, you can manage both your cloud infrastructure and Kubernetes workloads with the same tools.
import * as pulumi from "@pulumi/pulumi";
import * as kubernetes from "@pulumi/kubernetes";
// Create a K8s namespace.
const devNamespace = new kubernetes.core.v1.Namespace("devNamespace", {
metadata: {
name: "dev",
},
});
// Deploy the K8s nginx-ingress Helm chart into the created namespace.
const nginxIngress = new kubernetes.helm.v3.Chart("nginx-ingress", {
chart: "nginx-ingress",
namespace: devNamespace.metadata.name,
fetchOpts:{
repo: "https://charts.helm.sh/stable/",
},
});
import pulumi_kubernetes as kubernetes
# Create a K8s namespace.
dev_namespace = kubernetes.core.v1.Namespace(
"devNamespace",
metadata={
"name": "dev",
})
# Deploy the K8s nginx-ingress Helm chart into the created namespace.
nginx_ingress = kubernetes.helm.v3.Chart(
"nginx-ingress",
kubernetes.helm.v3.ChartOpts(
chart="nginx-ingress",
namespace=dev_namespace.metadata["name"],
fetch_opts=kubernetes.helm.v3.FetchOpts(
repo="https://charts.helm.sh/stable/",
),
),
)
package main
import (
corev1 "github.com/pulumi/pulumi-kubernetes/sdk/v2/go/kubernetes/core/v1"
"github.com/pulumi/pulumi-kubernetes/sdk/v2/go/kubernetes/helm/v3"
metav1 "github.com/pulumi/pulumi-kubernetes/sdk/v2/go/kubernetes/meta/v1"
"github.com/pulumi/pulumi/sdk/v2/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
// Create a K8s namespace.
ns, err := corev1.NewNamespace(ctx, "devNamespace", &corev1.NamespaceArgs{
Metadata: &metav1.ObjectMetaArgs{
Name: pulumi.String("dev"),
},
})
if err != nil {
return err
}
// Deploy the K8s nginx-ingress Helm chart into the created namespace.
_, err = helm.NewChart(ctx, "nginx-ingress", helm.ChartArgs{
Chart: pulumi.String("nginx-ingress"),
Namespace: ns.Metadata.ApplyT(func(metadata interface{}) string {
return *metadata.(*metav1.ObjectMeta).Name
}).(pulumi.StringOutput),
FetchArgs: helm.FetchArgs{
Repo: pulumi.String("https://charts.helm.sh/stable/"),
},
})
if err != nil {
return err
}
return nil
})
}
using Pulumi;
using Kubernetes = Pulumi.Kubernetes;
class MyStack : Stack
{
public MyStack()
{
// Create a K8s namespace.
var devNamespace = new Kubernetes.Core.V1.Namespace("devNamespace", new Kubernetes.Types.Inputs.Core.V1.NamespaceArgs
{
Metadata = new Kubernetes.Types.Inputs.Meta.V1.ObjectMetaArgs
{
Name = "dev",
},
});
// Deploy the K8s nginx-ingress Helm chart into the created namespace.
var nginx = new Kubernetes.Helm.V3.Chart("nginx-ingress", new Kubernetes.Helm.ChartArgs
{
Chart = "nginx-ingress",
Namespace = devNamespace.Metadata.Apply(x => x.Name),
FetchOptions = new Kubernetes.Helm.ChartFetchArgs
{
Repo = "https://charts.helm.sh/stable/"
},
});
}
}
package com.pulumi.example.infra;
import com.pulumi.Context;
import com.pulumi.Exports;
import com.pulumi.Pulumi;
import com.pulumi.kubernetes.core_v1.Namespace;
import com.pulumi.kubernetes.core_v1.NamespaceArgs;
import com.pulumi.kubernetes.meta_v1.inputs.ObjectMetaArgs;
import com.pulumi.kubernetes.helm.sh_v3.Release;
import com.pulumi.kubernetes.helm.sh_v3.ReleaseArgs;
import com.pulumi.kubernetes.helm.sh_v3.inputs.RepositoryOptsArgs;
public class Main {
public static void main(String[] args) {
Pulumi.run(Main::stack);
}
private static Exports stack(Context ctx) {
var devNamespace = new Namespace("devNamespace", NamespaceArgs.builder()
.metadata(ObjectMetaArgs.builder()
.name("dev")
.build())
.build());
var nginxIngress = new Release("nginx-ingress", ReleaseArgs.builder()
.chart("nginx-ingress)
.namespace(devNamespace.metadata.name)
.repositoryOpts(RepositoryOptsArgs.builder()
.repo("https://charts.helm.sh/stable/")
.build())
.build());
return ctx.exports();
}
}
name: simple-kubernetes
runtime: yaml
resources:
devNamespace:
type: kubernetes:core:Namespace
properties:
metadata:
name: "dev"
nginxIngress:
type: kubernetes:helm.sh:Chart
properties:
chart: "nginx-ingress"
namespace: ${devNamespace.metadata.name}
fetchOpts:
repo: "https://charts.helm.sh/stable/"
Run On Any Cloud
Support for all clouds including Amazon Elastic Kubernetes Service (EKS), Azure Kubernetes Service (AKS), Google Kubernetes Engine (GKE), DigitalOcean Kubernetes (DOKS), and more, with hundreds of integrations with popular infrastructure service providers.
Intelligent Cloud Management
Gain security, compliance, and cost insights into the entirety of an organization’s Kubernetes applications and cloud assets and automatically remediate issues through AI-powered workflows.
Automate Delivery
You can integrate Pulumi directly with your favorite CI/CD systems and testing frameworks to continuously deliver Kubernetes infrastructure and applications. Improve the velocity and visibility into your deployments from simple to complex global environments.
Smart Architecture
YAML and templated DSLs force you to write the same boilerplate code over and over. Pulumi’s Kubernetes library allows you to codify those patterns and best practices so you can stop reinventing the wheel and start inventing the platforms of the future.
Be Proactive, Not Reactive
When you enable Pulumi’s Policy as Code feature, you instantly gain the power to prevent mistakes from being deployed. Enforce security, compliance, cost controls, and best practices using policies defined in modern languages.
Centralize Secrets
Pulumi ESC is integrated with External Secrets Operator (ESO), enabling the passing of secrets from ESC directly as environment variables of applications running in Kubernetes.
Continue using the tools you love
Pulumi has first-class support for popular Kubernetes tools, such as Helm, Kustomize, YAML, Secret Managers, Open Policy Agent (OPA), Custom Resource Definitions (CRDs), and Server-Side Apply (SSA).
Learn MoreEverything In One Place
Easily make the best use of existing Kubernetes tools such as Helm, and reduce the friction caused by multiple deployment tools and models across complex architectures.
Efficient Adoption
There’s no need to rewrite your existing Kubernetes configurations to get started with Pulumi. You can efficiently adopt existing K8s resources to deploy your application to save time and effort.
Secrets Management
Use Pulumi to ensure secret data is encrypted in transit, at rest, and physically anywhere it gets stored. Bring your own preferred cloud encryption provider or use Pulumi’s native secrets provider.
Pulumi Kubernetes Operator
Deploy both Kubernetes resources and cloud infrastructure from within the Kubernetes resource model using a GitOps workflow. Use Pulumi’s Flux and ArgoCD integrations along with many other CI/CD integrations.
AI-powered Kubernetes Management
Pulumi Copilot, an AI-powered assistant, makes discovering cost savings, running compliance checks, and debugging deployments across your Kubernetes resources as easy as typing a question.
Learn MoreDiscover Cost Savings
Pulumi Copilot can access infrastructure stack and resource data, so you can analyze the cost of your infrastructure and reclaim cloud waste.
Run Compliance Checks
Pulumi Copilot leverages knowledge about compliance frameworks to analyze your infrastructure and check for policy compliance.
Debug Cloud Failures
Pulumi Copilot can access update and deployment logs of your stacks as well as access history, logs, and runtime metrics, so you can easily debug deployment and infrastructure failures.
Stay Secure
Pulumi Copilot combines Pulumi’s supergraph of your cloud infrastructure and knowledge about security best practices to identify security violations and and detect anomalous activity.
Get Started with Pulumi
Use Pulumi's open source SDK to create, deploy, and manage infrastructure on any cloud.