Trusted by your peers
Build and ship faster using
open source infrastructure as code
Author infrastructure code using programming languages you know and love. Write statements to define infrastructure using your IDE with autocomplete, type checking, and documentation.
“Our developers needed a fast, modular, and testable platform for managing cloud infrastructure. Nothing is better than having standard programming languages for building and managing infrastructure”
Austin Byers, Principal Platform Engineer
Manage configuration and secrets with modular Environments
Easy-to-use single source of truth for all configurations with guardrails. Seamlessly adopt short-lived dynamic secrets. Never have downtime over changed configurations because you can change once and have it updated everywhere. Enforce least-privileged access through role-based access controls.
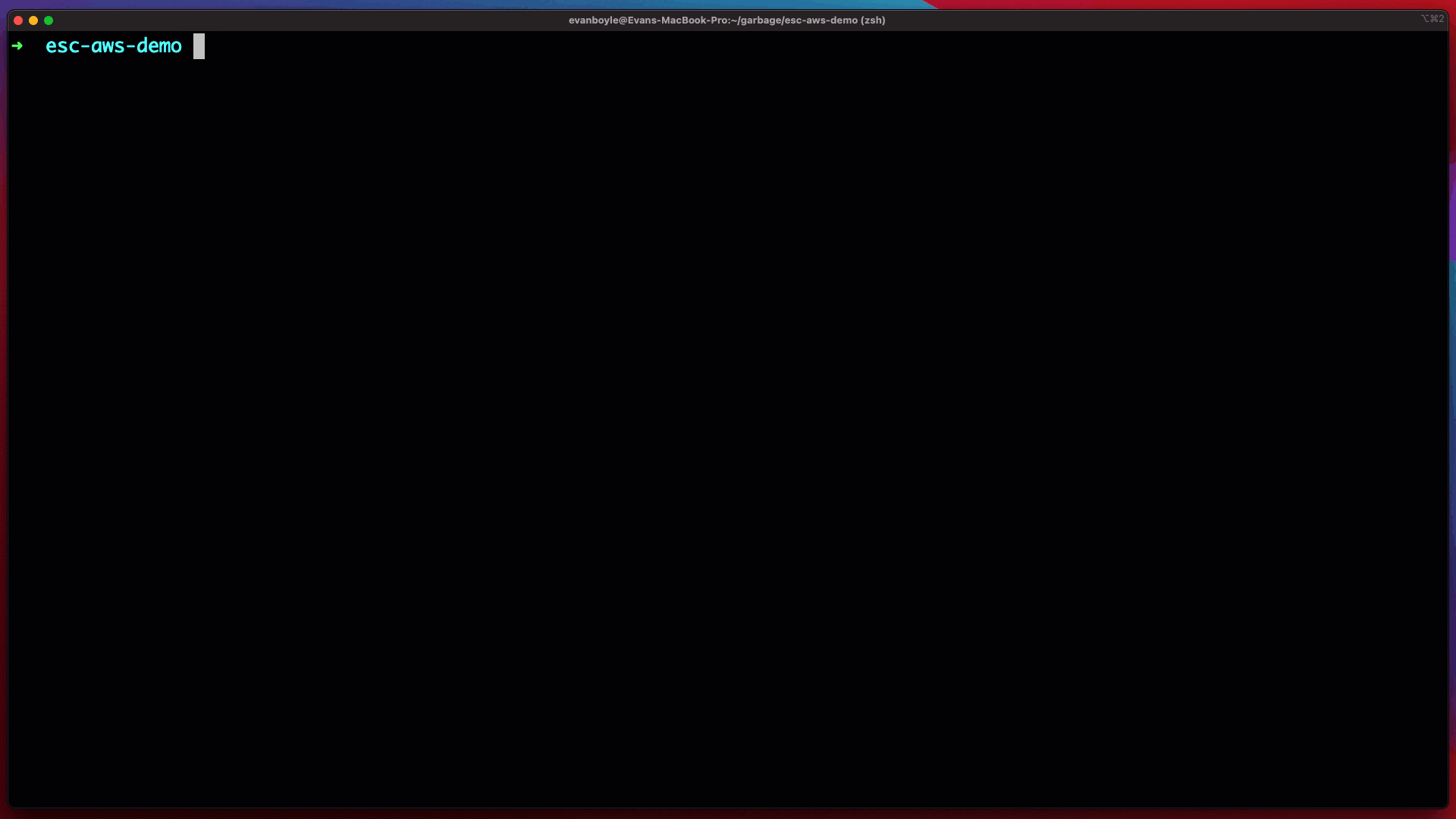
“Pulumi let us build and automate cloud infrastructure projects at a scale that simply wasn’t imaginable using prior-generation infrastructure as code technologies”
Matt Stephenson, Senior Principal Software Engineer
Build productive platforms for both developers and operators
Internal developer portals (IDPs) enable your developers to quickly provision security-compliant infrastructure, boost productivity with pre-configured architectures and automate testing and deployments, adhering to organizational standards.
Pulumi’s infrastructure and policy as code engine fosters collaboration between your developers, security, and operation teams through common, popular programming languages.
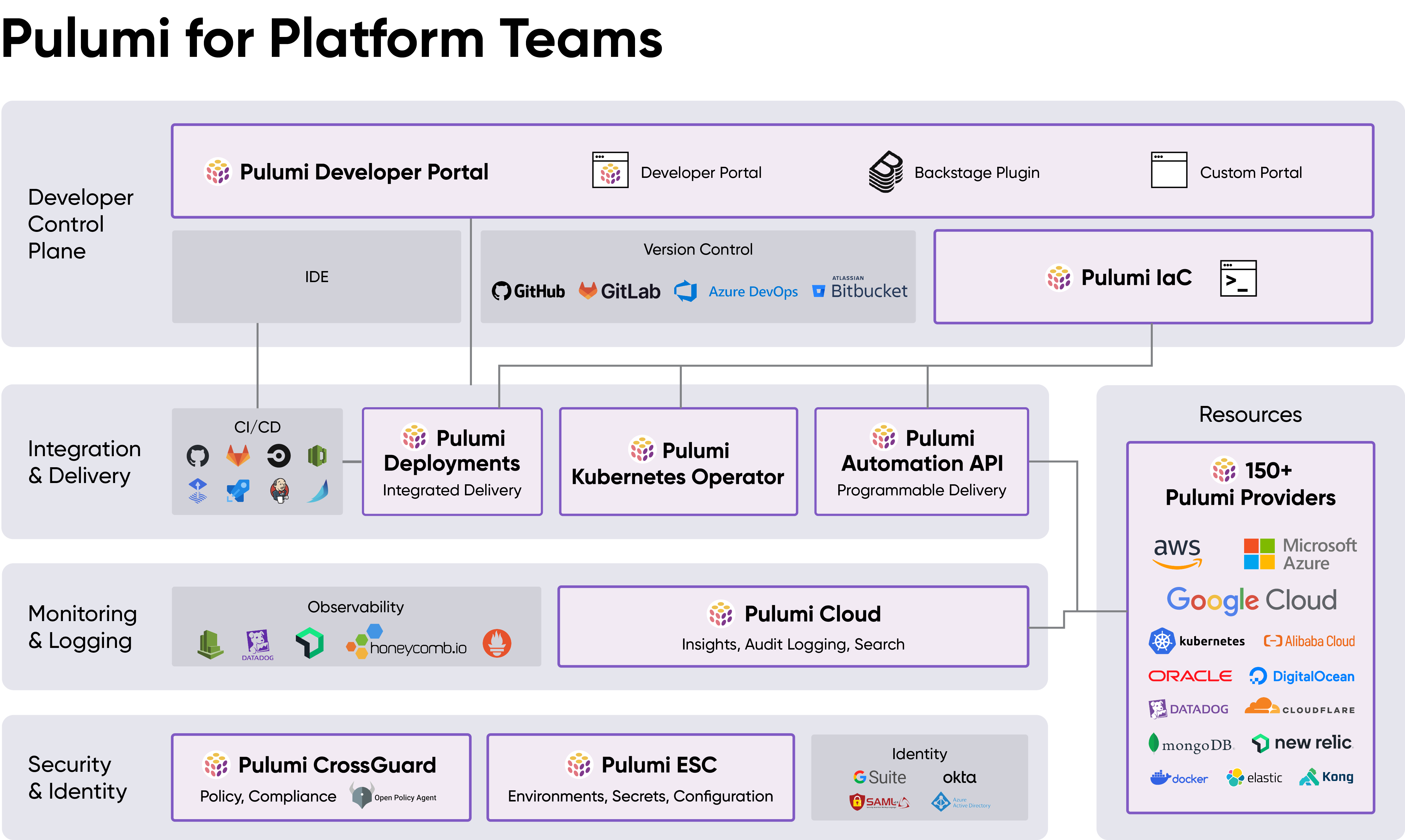
“Pulumi helped our team to ship a new product faster. We needed one tool to setup and manage multi-cloud, multi-region Kubernetes clusters that infrastructure and applications teams could use collaboratively”
Justin Fitzhugh, VP of Cloud Platform Engineering
Code on demand with Pulumi AI
Want help writing infrastructure code? Use Pulumi AI to generate code for your desired infrastructure – all through natural language commands.
Ask Pulumi AI to iterate on your code to make changes and add new resources.
Built by engineers for engineers. Open source.
and let’s build together.
There is no way around the fact that devops is complicated but @PulumiCorp is a game changer for me. Blows away CloudForamtion, TerraForm, CDK, etc.
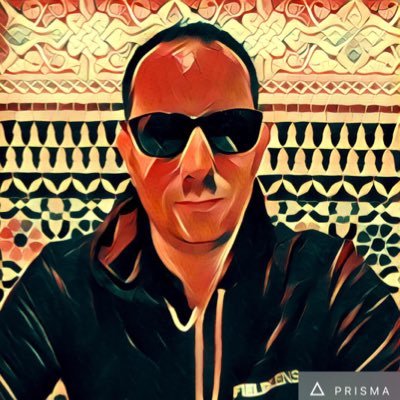
@BryanMigliorisi
Continuing on my thread about @PulumiCorp from a while ago: holy shit I am a convert. I needed to setup a staging environment that was mostly identical to prod, and once I trued up our Pulumi stack with AWS, it took *minutes* to do this. How have I lived without this until now?
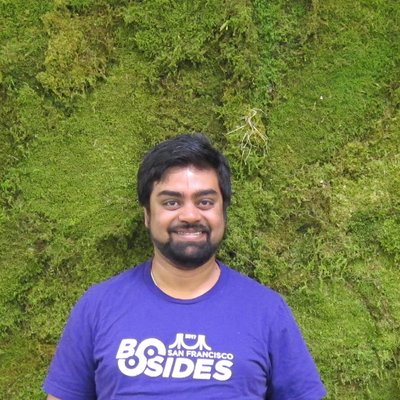
@krangarajan
Without a doubt the most approachable tool in the IaaC space is @PulumiCorp. Somewhat enjoying provisioning a scheduled run of a Lambda.
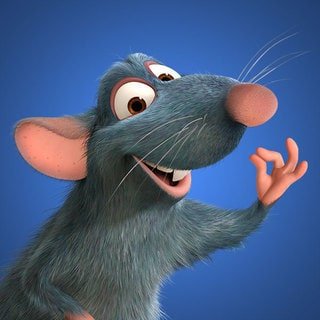
@Vetium
The developer experience of Pulumi is just sublime. As a prior Terraform user, the grass is substantially greener on this side. I'm so glad I made the switch two years back. Using Terraform for my current use case would be a massive downgrade.
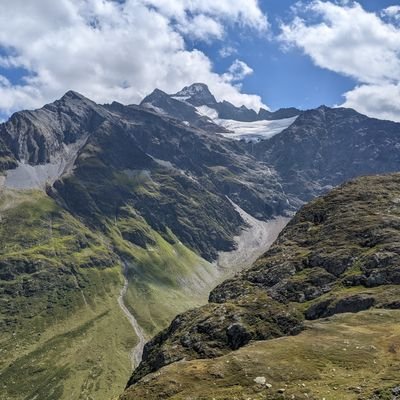
@justedagain
Give Pulumi a shot and you will never look back @PulumiCorp
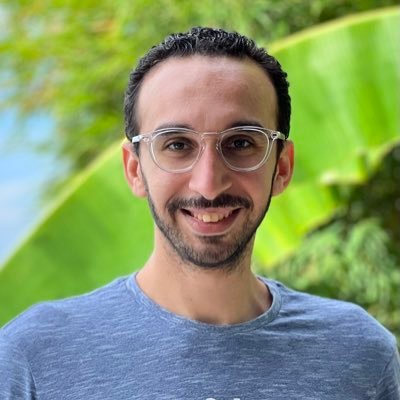
@hossambarakat_
With @PulumiCorp I said goodbye to #YAML and JSON supersets. I went back to what I love: #code. Code. End to end. Functional, even. #Kubernetes is pleasant again.
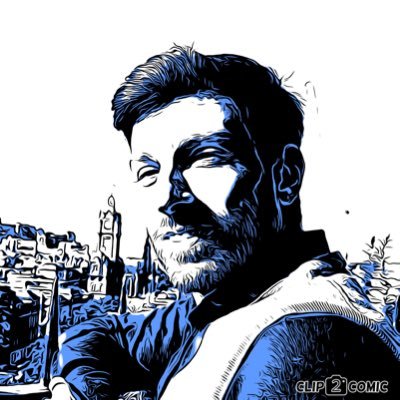
@matticala
Deploying cloud resources using @PulumiCorp is just amazing. Why would anybody bother with JSON, YAML or some other DSL?
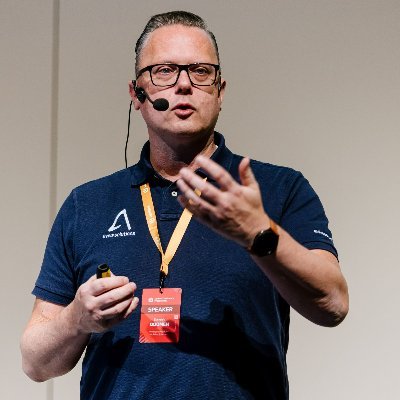
@ddoomen
Been using Pulumi with Typescript for a IaaC managing k8s and stateful databases. Don't see myself going back to using terraform after this.
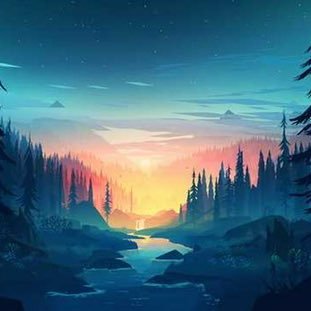
@Meliora245
our team at @devseed is now gravitating toward using https://pulumi.com/docs/concepts/vs/terraform/ instead of terraform because it's all in python so it is easier to onboard new people to the tool and makes it easier to manage the same infra definition in different test, staging, and deploy envs.
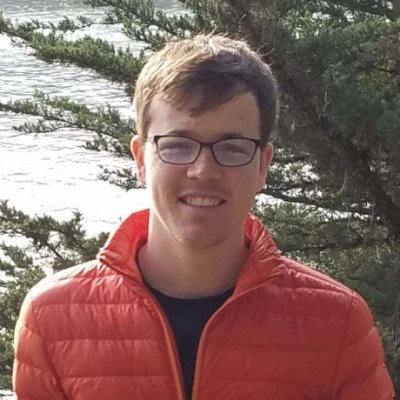
@rybavery
It wouldn't have been possible to build Sparky without @PulumiCorp. Shout out to the team and community for helping us get up and running!
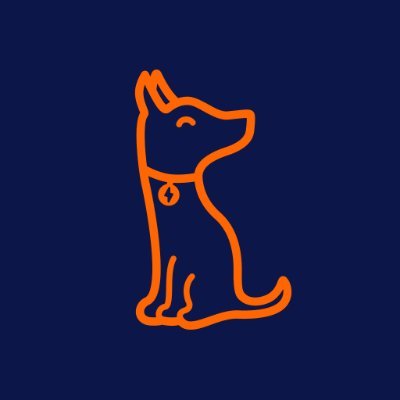
@SparkyCodes
ok so pulumi is awesome. almost no clicking, just scripting go and up
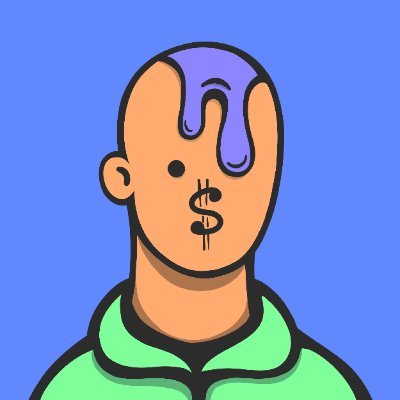
@0xksure
New gig uses @PulumiCorp to manage AWS infra. Initially I was skeptical and was tempted to go back to Terraform, but after using pulumi imports and discovering the ability to write tests easily, I'm a convert. (1/4)
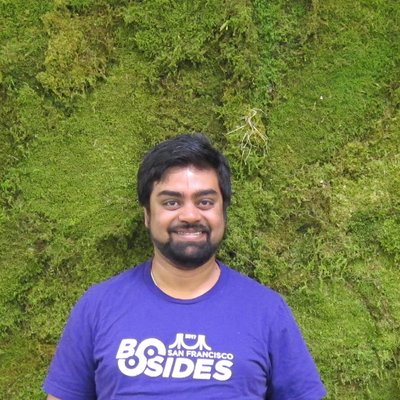
@krangarajan
I love @PulumiCorp so much because it is like 50% of the reason why we dared to build https://planton.cloud. An equivalent of 50K lines of declarative infra code has been put behind APIs to support the features on the platform with https://www.pulumi.com/docs/using-pulumi/automation-api/
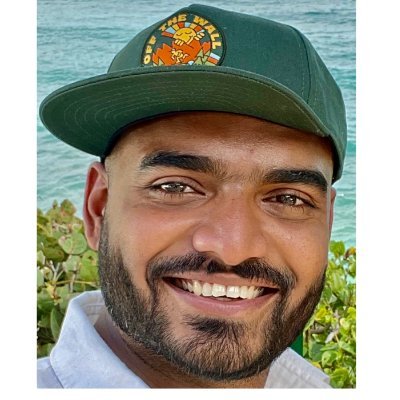
@swarupdonepudi
Today is a good day. Finished no-code website builder. Managed to automate deploying a website created with said builder with @PulumiCorp
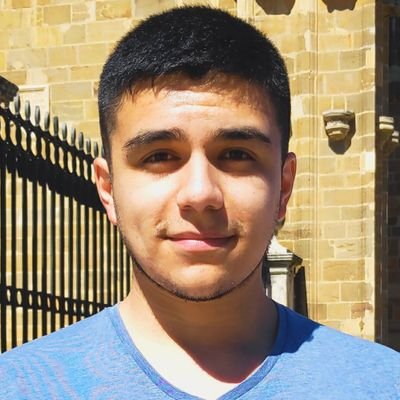
@iamjmoa
Ready to try Pulumi?
Follow the step-by-step guide
Download our open source SDK